参考std::string,设计并实现MyString类。 基本功能要求: 1)四种构造函数、析构函数; 2)重载赋值操作符=,使其正确完成String类型的赋值操作; 3)重载必要的操作符,实现形如 String a = “Hello ”; a += “World!”;的功能。 4)重载必要的操作符,实现形如 String a, b, c; c = a + b; 的操作过程。 5)重载必要的操作符,当完成 String a(“Hello ”); a << “world”; 的操作过程后,a所代表的字符串为”Hello world”。 6)重载必要的操作符,当完成 String a(“test”); std::cout << a; 的操作过程后,在屏幕上输入 test 7)重载必要的操作符,当完成 String a(“test”); a[2] = ’S’; 后,a所代表的字符串为”teSt”。
时间: 2024-02-03 07:02:41 浏览: 32
以下是MyString类的实现:
```cpp
#include <cstring>
#include <iostream>
class MyString {
public:
// 构造函数
MyString() : len(0), str(nullptr) {}
MyString(const char* s) : len(strlen(s)), str(new char[len + 1]) {
strcpy(str, s);
}
MyString(const MyString& rhs) : len(rhs.len), str(new char[len + 1]) {
strcpy(str, rhs.str);
}
MyString(MyString&& rhs) noexcept : len(rhs.len), str(rhs.str) {
rhs.len = 0;
rhs.str = nullptr;
}
// 析构函数
~MyString() {
delete[] str;
}
// 重载赋值操作符
MyString& operator=(const MyString& rhs) {
if (this != &rhs) {
delete[] str;
len = rhs.len;
str = new char[len + 1];
strcpy(str, rhs.str);
}
return *this;
}
// 重载+=操作符
MyString& operator+=(const MyString& rhs) {
char* new_str = new char[len + rhs.len + 1];
strcpy(new_str, str);
strcpy(new_str + len, rhs.str);
delete[] str;
str = new_str;
len += rhs.len;
return *this;
}
// 重载+操作符
friend MyString operator+(const MyString& lhs, const MyString& rhs) {
MyString res(lhs);
res += rhs;
return res;
}
// 重载<<操作符
friend std::ostream& operator<<(std::ostream& os, const MyString& rhs) {
os << rhs.str;
return os;
}
// 重载[]操作符
char& operator[](int i) {
return str[i];
}
// 重载<<操作符
MyString& operator<<(const char* s) {
int new_len = strlen(str) + strlen(s);
char* new_str = new char[new_len + 1];
strcpy(new_str, str);
strcpy(new_str + len, s);
delete[] str;
str = new_str;
len = new_len;
return *this;
}
private:
int len;
char* str;
};
```
这个实现中,我们使用了动态内存分配来存储字符串,这就需要我们自己来实现析构函数、拷贝构造函数、移动构造函数和赋值操作符,以防止内存泄漏等问题。另外,我们还重载了+=、+、[]、<<、>>等操作符,使得MyString类的使用更加方便。
相关推荐
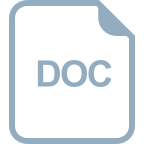










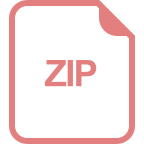