void Sift(int R[],int low,int high) { int i=low,j=2*i; int temp=R[i]; while (j<=high) { if (j<high && R[j]<R[j+1]) j++; if (temp<R[j]) { R[i]=R[j]; i=j; j= ; } else break; } R[i]= ; } void HeapSort(int R[],int n) { int i,j; int temp; for (i= ;i>=1;i--) Sift(R,i,n); for (i=n;i>=2;i--) { swap(R[1],R[i]); //将R[1]与R[i]进行交换。 ; } }
时间: 2024-02-14 10:21:48 浏览: 51
这段代码是一个基于堆排序的排序算法。其中Sift函数是用来对堆进行调整的,HeapSort函数是主函数。
在Sift函数中,参数R表示待排序的数组,low和high表示当前堆中需要调整的范围。变量i和j分别表示当前节点和它的左孩子节点,temp保存当前节点的值。循环中,如果右孩子比左孩子大,则选择右孩子作为比较对象;如果比较对象比当前节点大,则将比较对象赋值给当前节点,然后i更新为j,j更新为新的左孩子节点,继续循环,直到当前节点比它的左右孩子都大为止。最后将temp赋值给R[i],完成一次调整。
在HeapSort函数中,首先使用Sift函数将整个数组构建成一个堆。然后从后往前遍历数组,每次将堆顶元素(即最大值)与当前遍历到的元素进行交换,然后将堆的范围缩小1,并重新调整堆,直到数组有序。
相关问题
#include <stdio.h> #include <stdlib.h> #define TRUE 1 #define FALSE 0 typedef int KeyType; typedef int OtherType; typedef struct { KeyType key; OtherType other_data; }RecordType; void InsSort(RecordType r[], int length) { int i,j; for (i=2; i<=length; i++) { r[0]=r[i]; j=i-1; while (r[0].key< r[j].key ) { r[j+1]= r[j]; j=j-1; } r[j+1]=r[0]; } } void sift(RecordType r[], int k, int m) { RecordType t; int i,j; int x; int finished; t= r[k]; x=r[k].key; i=k; j=2*i; finished=FALSE; while( j<=m && !finished ) { if (j<m && r[j].key< r[j+1].key ) j=j+1; if ( x>= r[j].key) finished=TRUE; else { r[i] = r[j]; i=j; j=2*i; } } r[i] =t; } void crt_heap(RecordType r[], int length ) { int i,n; n= length; for ( i=n/2; i >= 1; --i) sift(r,i,n); } void HeapSort(RecordType r[],int length) { int i,n; RecordType b; crt_heap(r, length); n= length; for ( i=n ; i>= 2; --i) { b=r[1]; r[1]= r[i]; r[i]=b; sift(r,1,i-1); } } int main() { int i,j; RecordType r[20]; int len; printf("请输入待排序记录的长度:"); scanf("%d",&len); for(i=1;i<=len;i++) { printf("请输入第%d个记录元素:",i); fflush(stdin); scanf("%d",&j); r[i].key = j; } for(i=1;i<=len;i++) printf("%d ",r[i].key); printf("\n"); InsSort(r,len); for(i=1;i<=len;i++) printf("%d ",r[i].key); printf("\n"); HeapSort(r,len); for(i=1;i<=len;i++) printf("%d ",r[i].key); printf("\n"); return 0; }分别补充希尔排序和快速排序算法
补充希尔排序算法:
```c
void ShellSort(RecordType r[], int length) {
int i, j, gap;
RecordType temp;
for (gap = length / 2; gap > 0; gap /= 2) {
for (i = gap; i < length; i++) {
temp = r[i];
for (j = i - gap; j >= 0 && r[j].key > temp.key; j -= gap) {
r[j + gap] = r[j];
}
r[j + gap] = temp;
}
}
}
```
补充快速排序算法:
```c
int Partition(RecordType r[], int low, int high) {
RecordType pivot = r[low];
while (low < high) {
while (low < high && r[high].key >= pivot.key) {
high--;
}
r[low] = r[high];
while (low < high && r[low].key <= pivot.key) {
low++;
}
r[high] = r[low];
}
r[low] = pivot;
return low;
}
void QuickSort(RecordType r[], int low, int high) {
if (low < high) {
int pivotpos = Partition(r, low, high);
QuickSort(r, low, pivotpos - 1);
QuickSort(r, pivotpos + 1, high);
}
}
```
#include<stdio.h> #define FALSE 0 #define TRUE 1 typedef int KeyType,OtherType; typedef struct{ KeyType key; OtherType other_data; }RecordType; void print(RecordType r[],int len){ for(int i=1;i<=len;i++){ printf("%d ",r[i].key); }printf("\n"); } void sift(RecordType r[],int k,int m); void crt_heap(RecordType r[],int n){ for(int i=n/2;i>=1;i--) sift(r,i,n); } void sift(RecordType r[],int k,int m){ RecordType t=r[k]; int x=r[k].key; int i=k; int j=2*i; int finished=FALSE; while(j<=m&&!finished){ if(j+1<=m&&r[j].key<r[j+1].key) j++; if(x>=r[j].key) finished=TRUE; else{ r[i]=r[j]; i=j; j=2*i; } }r[i]=t; } void HeapSort(RecordType r[],int n){ crt_heap(r,n); RecordType b; for(int i=n;i>=2;i--){ b=r[1]; r[1]=r[i]; r[i]=b; sift(r,1,i-1); print(r,n); } } int main(){ RecordType r[100]; int m,i=1; while(1){ scanf("%d",&m); if(r[i].key==0)break; r[i].key=m; i++; }int n=i-1; HeapSort(r,n); return 0; }为什么没有输出
这段代码没有输出是因为在输入数据时,没有给定结束输入的条件。在 `main` 函数中,通过 `while(1)` 循环读入数据,但是没有在循环体中设置结束条件,导致程序一直在等待输入,无法进行下一步操作。
你可以在输入数据时,增加一个结束输入的标志,例如输入 `-1` 作为结束标志。同时,在 `while` 循环中添加一个判断,当读入数据为 `-1` 时,跳出循环。修改后的代码如下:
```c
int main() {
RecordType r[100];
int m, i = 1;
while (1) {
scanf("%d", &m);
if (m == -1) break; // 输入 -1 作为结束标志
r[i].key = m;
i++;
}
int n = i - 1;
HeapSort(r, n);
return 0;
}
```
这样,当输入 `-1` 时,程序会跳出循环,开始进行排序,并输出排序结果。
相关推荐
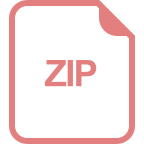
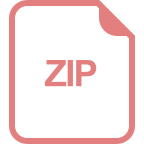












