设计一个复数Complex类,用友元函数重载乘法运算符(*),用成员函数重载除法运算符(/),完成两个复数对象的乘除法
时间: 2024-02-09 17:08:04 浏览: 29
好的,我可以帮你设计一个复数Complex类,并重载乘法和除法运算符。下面是这个类的代码实现:
```cpp
#include <iostream>
using namespace std;
class Complex {
private:
double real;
double imag;
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
friend Complex operator*(const Complex& c1, const Complex& c2);
Complex operator/(const Complex& c);
void display() { cout << real << " + " << imag << "i" << endl; }
};
Complex operator*(const Complex& c1, const Complex& c2) {
double r = c1.real * c2.real - c1.imag * c2.imag;
double i = c1.real * c2.imag + c1.imag * c2.real;
return Complex(r, i);
}
Complex Complex::operator/(const Complex& c) {
double denominator = c.real * c.real + c.imag * c.imag;
double r = (real * c.real + imag * c.imag) / denominator;
double i = (imag * c.real - real * c.imag) / denominator;
return Complex(r, i);
}
int main() {
Complex c1(1, 2);
Complex c2(3, 4);
Complex c3 = c1 * c2;
Complex c4 = c1 / c2;
c3.display();
c4.display();
return 0;
}
```
在上面的代码中,我们定义了一个Complex类,包含了实部和虚部两个私有成员变量。我们用一个构造函数来初始化这两个成员变量。然后,我们用`friend`关键字声明一个友元函数`operator*`,用来重载乘法运算符。这个函数接受两个Complex对象作为参数,返回它们的乘积。
接下来,我们用一个成员函数`operator/`来重载除法运算符。这个函数接受一个Complex对象作为参数,表示除数。它首先计算出除数的实部和虚部的平方和,然后用公式计算出商的实部和虚部,并返回一个新的Complex对象。
最后,我们在主函数中创建两个Complex对象,分别用它们的乘法和除法运算符来计算它们的乘积和商,并输出结果。
希望这个例子能够帮助你理解如何用友元函数和成员函数来重载运算符。如果你还有其他问题,请随时问我。
相关推荐
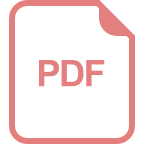
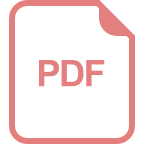
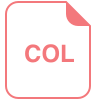
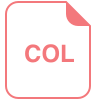
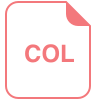
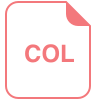






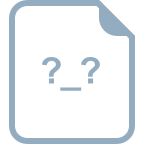
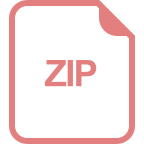