如何用java实现redis的read_through
时间: 2024-03-20 17:40:26 浏览: 53
"Read through" 是指在缓存没有命中时,从数据库中读取数据并将其添加到缓存中。在Java中实现Redis的"Read through"可以使用Redisson客户端库和Spring Cache抽象层。具体步骤如下:
1. 引入Redisson和Spring Cache的依赖包。
2. 在Spring配置文件中配置Redisson客户端连接信息。
3. 在Java代码中使用Spring Cache注解,例如@Cacheable和@CachePut注解,来标识需要缓存的方法,并指定缓存名称和缓存Key。
4. 在方法实现中,先从缓存中获取数据,如果缓存命中,则直接返回缓存数据。如果缓存未命中,则从数据库中查询数据,并将其添加到缓存中。
示例代码:
```
// 引入Redisson和Spring Cache的依赖包
<dependency>
<groupId>org.redisson</groupId>
<artifactId>redisson</artifactId>
<version>3.15.5</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
// 在Spring配置文件中配置Redisson客户端连接信息
@Bean(destroyMethod = "shutdown")
public RedissonClient redisson() {
Config config = new Config();
config.useSingleServer().setAddress("redis://127.0.0.1:6379");
return Redisson.create(config);
}
// 在Java代码中使用Spring Cache注解,例如@Cacheable和@CachePut注解,来标识需要缓存的方法,并指定缓存名称和缓存Key
@Cacheable(value = "userCache", key = "#id")
public User getUserById(Long id) {
// 在方法实现中,先从缓存中获取数据,如果缓存命中,则直接返回缓存数据。
User user = getUserFromCache(id);
if (user != null) {
return user;
}
// 如果缓存未命中,则从数据库中查询数据,并将其添加到缓存中
user = getUserFromDB(id);
if (user != null) {
cacheUser(id, user);
}
return user;
}
// 从缓存中获取数据
private User getUserFromCache(Long id) {
RMap<Long, User> userCache = redissonClient.getMap("userCache");
return userCache.get(id);
}
// 将数据添加到缓存中
private void cacheUser(Long id, User user) {
RMap<Long, User> userCache = redissonClient.getMap("userCache");
userCache.put(id, user);
}
// 从数据库中查询数据
private User getUserFromDB(Long id) {
// ...
}
```
阅读全文
相关推荐
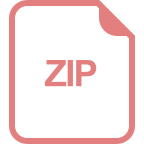
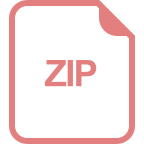
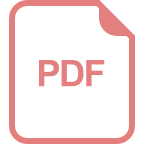
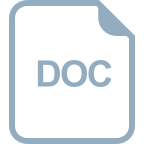
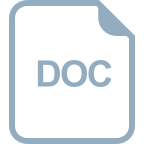
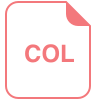
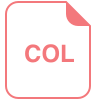
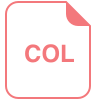
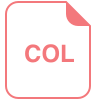
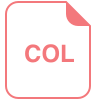
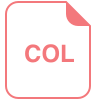

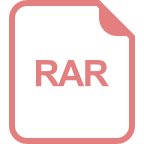
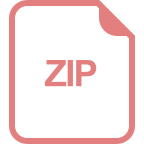