有向图G采用邻接表存储,判断给定两个顶点a、b之间是否存在一条从a到b的路径。使用c++实现代码
时间: 2024-03-24 11:40:26 浏览: 61
可以使用深度优先搜索(DFS)或广度优先搜索(BFS)来判断两个顶点之间是否存在路径。以下是使用DFS的C++代码示例:
```c++
#include <iostream>
#include <vector>
#include <stack>
using namespace std;
// 有向图采用邻接表存储
class Graph {
public:
Graph(int n) {
adjList.resize(n);
}
// 添加边
void addEdge(int u, int v) {
adjList[u].push_back(v);
}
// 判断是否存在从s到t的路径
bool hasPath(int s, int t) {
vector<bool> visited(adjList.size(), false); // 记录节点是否被访问过
stack<int> st;
st.push(s);
visited[s] = true;
while (!st.empty()) {
int u = st.top();
st.pop();
for (int v : adjList[u]) {
if (v == t) {
return true; // 找到从s到t的路径
}
if (!visited[v]) {
st.push(v);
visited[v] = true;
}
}
}
return false; // 没有找到从s到t的路径
}
private:
vector<vector<int>> adjList;
};
int main() {
Graph g(5);
g.addEdge(0, 1);
g.addEdge(1, 2);
g.addEdge(2, 3);
g.addEdge(3, 4);
cout << g.hasPath(0, 4) << endl; // 输出1,即存在从0到4的路径
cout << g.hasPath(4, 0) << endl; // 输出0,即不存在从4到0的路径
return 0;
}
```
在上述代码中,我们使用了深度优先搜索(DFS)来遍历图中的节点,并且使用一个栈来保存待访问的节点。在遍历过程中,我们记录每个节点是否被访问过,避免重复访问。如果找到了从s到t的路径,我们就返回true。如果遍历完所有的节点都没有找到从s到t的路径,我们就返回false。
阅读全文
相关推荐
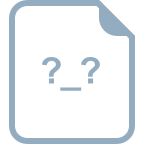
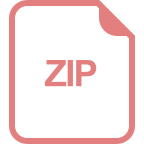













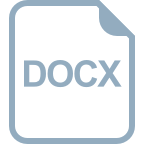
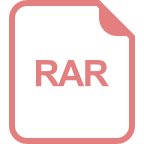
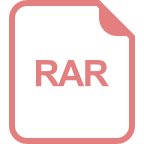