题目描述 利用重载运算符,实现矩阵的加法,减法。 请重载加减运算符并自定义矩阵类完成相应的操作 输入 第一行为测试数据数 对于每组测试数据 第一行为矩阵的行数和列数 接下来分别为两个矩阵的各个元素 输出 输出矩阵的和与差
时间: 2023-11-27 17:53:42 浏览: 50
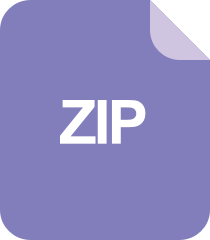
C++矩阵类的编写,基于运算符重载
以下是一个可能的实现,其中矩阵类使用动态分配的二维数组来存储矩阵元素:
```
#include <iostream>
using namespace std;
class Matrix {
private:
int rows;
int cols;
int **data;
public:
Matrix(int r, int c) {
rows = r;
cols = c;
data = new int*[rows];
for (int i = 0; i < rows; i++) {
data[i] = new int[cols];
}
}
Matrix(const Matrix& other) {
rows = other.rows;
cols = other.cols;
data = new int*[rows];
for (int i = 0; i < rows; i++) {
data[i] = new int[cols];
for (int j = 0; j < cols; j++) {
data[i][j] = other.data[i][j];
}
}
}
~Matrix() {
for (int i = 0; i < rows; i++) {
delete[] data[i];
}
delete[] data;
}
Matrix operator+(const Matrix& other) const {
if (rows != other.rows || cols != other.cols) {
throw "Matrix dimensions do not match!";
}
Matrix result(rows, cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
result.data[i][j] = data[i][j] + other.data[i][j];
}
}
return result;
}
Matrix operator-(const Matrix& other) const {
if (rows != other.rows || cols != other.cols) {
throw "Matrix dimensions do not match!";
}
Matrix result(rows, cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
result.data[i][j] = data[i][j] - other.data[i][j];
}
}
return result;
}
friend ostream& operator<<(ostream& os, const Matrix& mat) {
for (int i = 0; i < mat.rows; i++) {
for (int j = 0; j < mat.cols; j++) {
os << mat.data[i][j] << " ";
}
os << endl;
}
return os;
}
};
int main() {
int t;
cin >> t;
while (t--) {
int m, n;
cin >> m >> n;
Matrix A(m, n);
Matrix B(m, n);
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
cin >> A.data[i][j];
}
}
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
cin >> B.data[i][j];
}
}
try {
cout << A + B << endl;
cout << A - B << endl;
} catch (const char* msg) {
cerr << msg << endl;
}
}
return 0;
}
```
在主函数中,首先读入测试数据的数量。对于每组测试数据,读入矩阵的行数和列数,然后分别读入两个矩阵的元素。然后使用重载的加减运算符求出矩阵的和与差,并输出结果。如果矩阵的维度不匹配,则抛出异常。注意,这里使用了 `try` 和 `catch` 来捕获异常,以便程序能够正常结束。
阅读全文
相关推荐
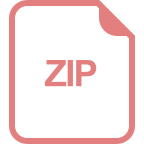















