设计一个矩阵类,c。要求:矩阵类中重载加(+)、减(一)、乘(*)、赋值(=)和加 赋值(+=)运算符。
时间: 2024-05-30 17:14:00 浏览: 227
可以的,我可以为您提供有关设计矩阵类的建议和指导。您可以在代码中添加如下操作符重载函数:
- 加法操作符重载函数:实现两个矩阵相加的操作。
- 减法操作符重载函数:实现两个矩阵相减的操作。
- 乘法操作符重载函数:实现两个矩阵相乘的操作。
- 赋值操作符重载函数:实现将一个矩阵赋值给另一个矩阵的操作。
- 加赋值操作符重载函数:实现将一个矩阵与另一个矩阵相加,并将结果赋值给原矩阵的操作。
您可以在这些函数中实现对应的矩阵操作,以达到您所需的需求。
相关问题
设计一个矩阵的要求重载加减乘赋值和加赋值
好的,这是一个关于编程的问题,我可以回答。 对于这个问题,您需要设计一个矩阵类,实现重载加减乘赋值和加赋值运算符。在设计时需要考虑以下要点:
1. 该类需要一个二维数组存储矩阵的值。
2. 重载运算符时需要注意运算对象的类型和维度。
3. 矩阵乘法需要保证左矩阵的列数等于右矩阵的行数。
4. 加、减法需要保证两个矩阵的维度相同。
实现方法可以参考现有的矩阵类库,例如Eigen库。
c语言写一个矩阵类cMatrix,基本要求如下:实现构造、拷贝构造与析构函数;支持任意大小的矩阵:行数和列数可以动态设置(不用修改代码)重载操作符*,实现矩阵相乘;重载操作符=,实现矩阵赋值;重载操作符++和--,分别实现矩阵元素同时+1和-1;在main函数中对以上功能进行调用
下面是一个简单的实现:
```c
#include <iostream>
using namespace std;
class cMatrix {
public:
// 构造函数
cMatrix(int n = 0, int m = 0) : rows(n), cols(m) {
data = new int*[rows];
for (int i = 0; i < rows; ++i) {
data[i] = new int[cols];
for (int j = 0; j < cols; ++j) {
data[i][j] = 0;
}
}
}
// 拷贝构造函数
cMatrix(const cMatrix& mat) {
rows = mat.rows;
cols = mat.cols;
data = new int*[rows];
for (int i = 0; i < rows; ++i) {
data[i] = new int[cols];
for (int j = 0; j < cols; ++j) {
data[i][j] = mat.data[i][j];
}
}
}
// 析构函数
~cMatrix() {
for (int i = 0; i < rows; ++i) {
delete[] data[i];
}
delete[] data;
}
// 矩阵相乘
cMatrix operator*(const cMatrix& mat) const {
if (cols != mat.rows) {
throw "The size of two matrices do not match!";
}
cMatrix result(rows, mat.cols);
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < mat.cols; ++j) {
for (int k = 0; k < cols; ++k) {
result.data[i][j] += data[i][k] * mat.data[k][j];
}
}
}
return result;
}
// 矩阵赋值
cMatrix& operator=(const cMatrix& mat) {
if (this != &mat) {
for (int i = 0; i < rows; ++i) {
delete[] data[i];
}
delete[] data;
rows = mat.rows;
cols = mat.cols;
data = new int*[rows];
for (int i = 0; i < rows; ++i) {
data[i] = new int[cols];
for (int j = 0; j < cols; ++j) {
data[i][j] = mat.data[i][j];
}
}
}
return *this;
}
// 矩阵元素同时+1
cMatrix& operator++() {
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
++data[i][j];
}
}
return *this;
}
// 矩阵元素同时-1
cMatrix& operator--() {
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
--data[i][j];
}
}
return *this;
}
// 输出矩阵
friend ostream& operator<<(ostream& os, const cMatrix& mat) {
for (int i = 0; i < mat.rows; ++i) {
for (int j = 0; j < mat.cols; ++j) {
os << mat.data[i][j] << " ";
}
os << endl;
}
return os;
}
private:
int rows;
int cols;
int** data;
};
int main() {
cMatrix mat1(2, 3);
mat1 = cMatrix(2, 3);
mat1 = cMatrix(3, 2);
cMatrix mat2(3, 4);
mat2 = cMatrix(4, 3);
cMatrix mat3 = mat1 * mat2;
cout << mat1 << endl;
cout << mat2 << endl;
cout << mat3 << endl;
++mat1;
cout << mat1 << endl;
--mat1;
cout << mat1 << endl;
return 0;
}
```
在这个例子中,我们首先定义了一个`cMatrix`类,并实现了构造、拷贝构造、析构函数以及运算符重载等功能。在`main`函数中,我们创建了两个矩阵`mat1`和`mat2`,并对它们进行赋值和相乘操作,最后输出结果。同时,我们也演示了如何使用运算符重载来实现矩阵元素同时加减一的操作。
阅读全文
相关推荐
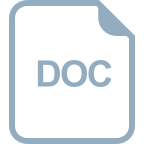
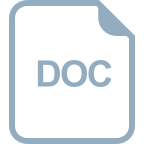
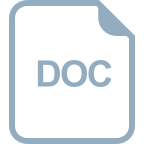













