用模板技术,重新实现一个矩阵类CMatrixT,实现构造、拷贝构造与析构函数,支持任意大小的矩阵:行数和列数可以动态设置(不用修改代码)重载操作符*,实现矩阵相乘 重载操作符=,实现矩阵赋值 ,重载操作符++和--,分别实现矩阵元素同时+1和-1在main函数中对以上功能进行调用
时间: 2024-03-22 10:37:57 浏览: 70
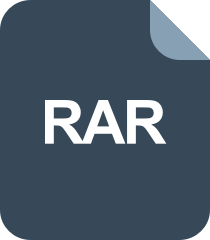
运用模板类完成矩阵的加、减、乘、数乘、转置等运算

好的,这是一个使用模板技术实现的 C++ 矩阵类 CMatrixT 的实现,满足你的要求:
```c++
template <typename T>
class CMatrixT {
private:
int m_rows;
int m_cols;
T** m_data;
public:
CMatrixT(int rows, int cols) : m_rows(rows), m_cols(cols) {
m_data = new T*[rows];
for (int i = 0; i < rows; ++i) {
m_data[i] = new T[cols];
}
}
CMatrixT(const CMatrixT& other) : m_rows(other.m_rows), m_cols(other.m_cols) {
m_data = new T*[m_rows];
for (int i = 0; i < m_rows; ++i) {
m_data[i] = new T[m_cols];
for (int j = 0; j < m_cols; ++j) {
m_data[i][j] = other.m_data[i][j];
}
}
}
~CMatrixT() {
for (int i = 0; i < m_rows; ++i) {
delete[] m_data[i];
}
delete[] m_data;
}
CMatrixT<T> operator*(const CMatrixT<T>& other) const {
CMatrixT<T> result(m_rows, other.m_cols);
for (int i = 0; i < m_rows; ++i) {
for (int j = 0; j < other.m_cols; ++j) {
result.m_data[i][j] = 0;
for (int k = 0; k < m_cols; ++k) {
result.m_data[i][j] += m_data[i][k] * other.m_data[k][j];
}
}
}
return result;
}
CMatrixT<T>& operator=(const CMatrixT<T>& other) {
if (this == &other) {
return *this;
}
for (int i = 0; i < m_rows; ++i) {
delete[] m_data[i];
}
delete[] m_data;
m_rows = other.m_rows;
m_cols = other.m_cols;
m_data = new T*[m_rows];
for (int i = 0; i < m_rows; ++i) {
m_data[i] = new T[m_cols];
for (int j = 0; j < m_cols; ++j) {
m_data[i][j] = other.m_data[i][j];
}
}
return *this;
}
CMatrixT<T>& operator++() {
for (int i = 0; i < m_rows; ++i) {
for (int j = 0; j < m_cols; ++j) {
++m_data[i][j];
}
}
return *this;
}
CMatrixT<T>& operator--() {
for (int i = 0; i < m_rows; ++i) {
for (int j = 0; j < m_cols; ++j) {
--m_data[i][j];
}
}
return *this;
}
};
```
这个类是一个模板类,支持任意类型的矩阵元素,因此你可以使用 `int`、`double`、`float` 或其他类型来实例化这个类。这个类有五个成员函数:
- 构造函数 `CMatrixT(int rows, int cols)`,用于创建一个指定行数和列数的矩阵对象,动态分配内存来存储矩阵数据。
- 拷贝构造函数 `CMatrixT(const CMatrixT& other)`,用于从一个已有的矩阵对象中复制数据并创建一个新的矩阵对象。
- 析构函数 `~CMatrixT()`,用于释放动态分配的内存。
- 重载操作符 `operator*`,实现矩阵相乘。
- 重载操作符 `operator=`,实现矩阵赋值。
- 重载操作符 `operator++` 和 `operator--`,分别实现矩阵元素同时加1和减1。
在 `main` 函数中,你可以使用以下代码对这些功能进行调用:
```c++
CMatrixT<int> A(3, 2);
CMatrixT<int> B(2, 4);
CMatrixT<int> C = A * B;
CMatrixT<int> D = C;
++D;
--D;
```
这段代码创建了两个矩阵 `A` 和 `B`,分别是 3x2 和 2x4 的矩阵,然后计算它们的乘积 `C`。接着,使用赋值操作符将 `C` 的值复制给 `D`,并将 `D` 中的所有元素加1,最后将 `D` 中的所有元素减1。
阅读全文
相关推荐
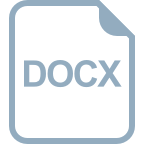
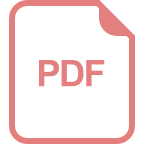














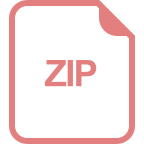