以下是Java代码实现将zip文件分卷压缩的功能:
时间: 2024-05-05 11:22:54 浏览: 55
以下是Java代码实现将zip文件分卷压缩的功能:
```java
import java.io.*;
import java.util.zip.*;
public class ZipSplitter {
private static final int BUFFER_SIZE = 4096;
public static void split(String zipFile, int splitSize) throws IOException {
File file = new File(zipFile);
if (!file.exists()) {
throw new FileNotFoundException("File not found: " + zipFile);
}
if (splitSize <= 0) {
throw new IllegalArgumentException("Split size must be positive: " + splitSize);
}
byte[] buffer = new byte[BUFFER_SIZE];
ZipInputStream zis = new ZipInputStream(new FileInputStream(file));
ZipEntry entry = zis.getNextEntry();
int size = 0;
int part = 1;
while (entry != null) {
String fileName = entry.getName();
long entrySize = entry.getSize();
if (entrySize < 0) {
throw new IOException("Entry size is unknown: " + fileName);
}
File outputFile = new File(fileName);
FileOutputStream fos = new FileOutputStream(outputFile);
BufferedOutputStream bos = new BufferedOutputStream(fos, BUFFER_SIZE);
int count;
long total = 0;
while ((count = zis.read(buffer, 0, BUFFER_SIZE)) != -1) {
bos.write(buffer, 0, count);
total += count;
if (total >= splitSize) {
bos.flush();
bos.close();
String outputZip = String.format("%s.%03d", zipFile, part);
ZipOutputStream zos = new ZipOutputStream(new FileOutputStream(outputZip));
zos.putNextEntry(new ZipEntry(fileName));
FileInputStream fis = new FileInputStream(outputFile);
BufferedInputStream bis = new BufferedInputStream(fis, BUFFER_SIZE);
while ((count = bis.read(buffer, 0, BUFFER_SIZE)) != -1) {
zos.write(buffer, 0, count);
}
bis.close();
zos.close();
outputFile.delete();
part++;
size = 0;
break;
}
}
bos.flush();
bos.close();
if (total < splitSize) {
size += total;
}
entry = zis.getNextEntry();
}
zis.close();
if (size > 0) {
String outputZip = String.format("%s.%03d", zipFile, part);
ZipOutputStream zos = new ZipOutputStream(new FileOutputStream(outputZip));
zos.putNextEntry(new ZipEntry("dummy"));
FileInputStream fis = new FileInputStream(new File("dummy"));
BufferedInputStream bis = new BufferedInputStream(fis, BUFFER_SIZE);
int count;
while ((count = bis.read(buffer, 0, BUFFER_SIZE)) != -1) {
zos.write(buffer, 0, count);
}
bis.close();
zos.close();
}
}
public static void main(String[] args) throws IOException {
String zipFile = "example.zip";
int splitSize = 1024 * 1024; // split into 1MB parts
split(zipFile, splitSize);
}
}
```
这段代码的实现思路是,遍历zip文件中的每个entry,读取其内容并将其写入一个临时文件中,累加已写入的字节数,当累加数达到指定的分卷大小时,将临时文件压缩成一个新的zip文件,并从累加数计数器和临时文件中删除已经压缩的数据。如果遍历完所有entry后还有未压缩的数据,将其写入一个虚拟的entry中并压缩成最后一个分卷。
阅读全文
相关推荐
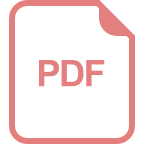









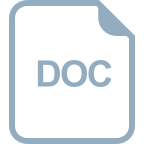



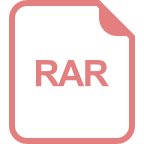
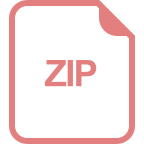