java判断大图是否包含小图
时间: 2023-10-02 21:10:12 浏览: 149
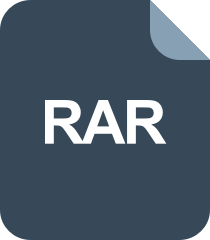
java实现大图查找小图

要判断一张大图是否包含一张小图,可以使用Java中的模板匹配。下面是一个基本的示例代码:
``` java
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
public class ImageProcessing {
public static void main(String[] args) throws IOException {
// 加载大图和小图
BufferedImage bigImg = ImageIO.read(new File("bigImg.jpg"));
BufferedImage smallImg = ImageIO.read(new File("smallImg.jpg"));
// 进行模板匹配
int result = matchTemplate(bigImg, smallImg);
// 输出匹配结果
if (result > 0) {
System.out.println("小图在大图中存在!");
} else {
System.out.println("小图在大图中不存在!");
}
}
public static int matchTemplate(BufferedImage bigImg, BufferedImage smallImg) {
int bigWidth = bigImg.getWidth();
int bigHeight = bigImg.getHeight();
int smallWidth = smallImg.getWidth();
int smallHeight = smallImg.getHeight();
// 初始化匹配结果
int result = 0;
// 遍历大图中每个像素
for (int y = 0; y < bigHeight - smallHeight; y++) {
for (int x = 0; x < bigWidth - smallWidth; x++) {
// 计算小图在大图中的匹配程度
int match = 0;
for (int i = 0; i < smallWidth; i++) {
for (int j = 0; j < smallHeight; j++) {
int bigPixel = bigImg.getRGB(x + i, y + j);
int smallPixel = smallImg.getRGB(i, j);
if (bigPixel == smallPixel) {
match++;
}
}
}
// 如果匹配程度达到阈值,说明小图在大图中存在
if (match > smallWidth * smallHeight * 0.8) {
result++;
}
}
}
return result;
}
}
```
在上面的示例代码中,我们使用 `ImageIO` 类加载大图和小图。然后,我们定义了一个 `matchTemplate` 函数,该函数使用嵌套循环遍历大图中的每个像素,并计算小图在该像素点的匹配程度。如果匹配程度达到阈值,则说明小图在大图中存在。最后,我们在 `main` 函数中调用 `matchTemplate` 函数,并输出匹配结果。
需要注意的是,上面示例代码中的模板匹配算法非常简单,只能处理一些简单的情况。如果要处理更加复杂的情况,可以使用更高级的模板匹配算法,比如 OpenCV 库中提供的算法。
阅读全文
相关推荐

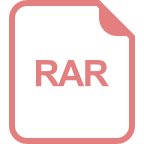
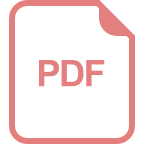
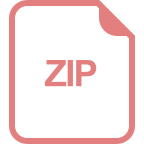
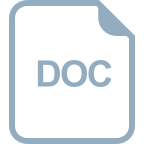
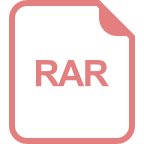
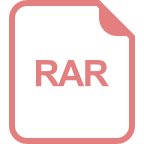
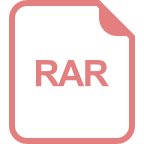
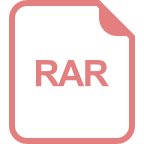
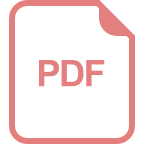
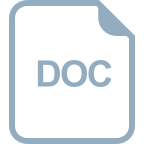
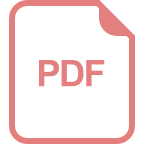
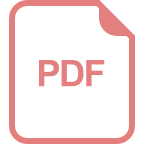
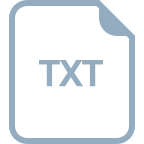
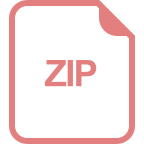