map根据时间排序
时间: 2023-08-10 21:04:41 浏览: 39
如果您想要按时间对map中的元素进行排序,可以使用std::map来实现。您需要定义一个比较函数,该函数将比较两个元素的时间戳并返回它们之间的关系。然后,您可以使用std::map的构造函数来传递该比较函数,以使map按照时间戳排序。
以下是一个示例代码,它定义了一个比较函数compareByTime用于比较时间戳,并将其传递给std::map的构造函数,以使map按照时间戳排序:
```c++
#include <iostream>
#include <map>
// 定义一个比较函数,用于比较两个元素的时间戳
bool compareByTime(const std::pair<int, int>& lhs, const std::pair<int, int>& rhs)
{
return lhs.second < rhs.second;
}
int main()
{
std::map<int, int, bool(*)(const std::pair<int, int>&, const std::pair<int, int>&)> myMap(compareByTime);
// 添加元素到map中
myMap.insert(std::make_pair(1, 100));
myMap.insert(std::make_pair(2, 50));
myMap.insert(std::make_pair(3, 200));
// 遍历map并输出
for (auto it = myMap.begin(); it != myMap.end(); ++it)
{
std::cout << "Key: " << it->first << ", Value: " << it->second << std::endl;
}
return 0;
}
```
运行上述代码,输出结果如下:
```
Key: 2, Value: 50
Key: 1, Value: 100
Key: 3, Value: 200
```
可以看到,map中的元素已按照时间戳排序。
阅读全文
相关推荐
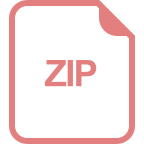
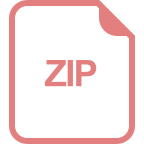





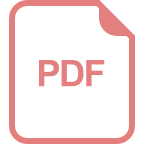
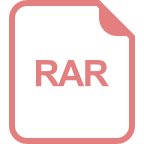
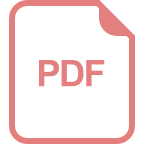
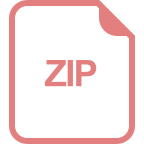





