用C语言实现陷波法进行啸叫抑制的代码
时间: 2024-03-15 21:45:46 浏览: 36
以下是使用C语言实现陷波法进行啸叫抑制的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdint.h>
#define BUFFER_SIZE 1024
typedef struct {
char ChunkID[4];
uint32_t ChunkSize;
char Format[4];
char Subchunk1ID[4];
uint32_t Subchunk1Size;
uint16_t AudioFormat;
uint16_t NumChannels;
uint32_t SampleRate;
uint32_t ByteRate;
uint16_t BlockAlign;
uint16_t BitsPerSample;
char Subchunk2ID[4];
uint32_t Subchunk2Size;
} WavHeader;
float b0 = 1.0, b1 = -1.0, a1 = -0.9;
float x1 = 0.0, y1 = 0.0;
int16_t apply_trap_filter(int16_t sample) {
float x = (float)sample / (float)INT16_MAX;
float y = b0 * x + b1 * x1 + a1 * y1;
x1 = x;
y1 = y;
float value = y * (float)INT16_MAX;
if (value > INT16_MAX) {
value = INT16_MAX;
} else if (value < INT16_MIN) {
value = INT16_MIN;
}
return (int16_t)value;
}
int main(int argc, char **argv) {
if (argc != 3) {
printf("Usage: %s [input_file] [output_file]\n", argv[0]);
return 1;
}
char *input_file = argv[1];
char *output_file = argv[2];
FILE *fp = fopen(input_file, "rb");
if (!fp) {
printf("Failed to open input file: %s\n", input_file);
return 1;
}
fseek(fp, 0, SEEK_END);
long file_size = ftell(fp);
fseek(fp, 0, SEEK_SET);
WavHeader header;
fread(&header, sizeof(header), 1, fp);
if (header.ChunkID[0] != 'R' || header.ChunkID[1] != 'I' || header.ChunkID[2] != 'F' || header.ChunkID[3] != 'F' ||
header.Format[0] != 'W' || header.Format[1] != 'A' || header.Format[2] != 'V' || header.Format[3] != 'E' ||
header.Subchunk1ID[0] != 'f' || header.Subchunk1ID[1] != 'm' || header.Subchunk1ID[2] != 't' || header.Subchunk1ID[3] != ' ' ||
header.Subchunk2ID[0] != 'd' || header.Subchunk2ID[1] != 'a' || header.Subchunk2ID[2] != 't' || header.Subchunk2ID[3] != 'a') {
printf("Invalid wav file format\n");
fclose(fp);
return 1;
}
uint32_t sample_rate = header.SampleRate;
uint16_t bits_per_sample = header.BitsPerSample;
uint32_t num_samples = header.Subchunk2Size / (bits_per_sample / 8) / header.NumChannels;
int16_t *samples = (int16_t *)malloc(num_samples * sizeof(int16_t));
fread(samples, sizeof(int16_t), num_samples, fp);
fclose(fp);
int16_t *filtered_samples = (int16_t *)malloc(num_samples * sizeof(int16_t));
for (int i = 0; i < num_samples; i++) {
filtered_samples[i] = apply_trap_filter(samples[i]);
}
free(samples);
fp = fopen(output_file, "wb");
if (!fp) {
printf("Failed to open output file: %s\n", output_file);
free(filtered_samples);
return 1;
}
fwrite(&header, sizeof(header), 1, fp);
fwrite(filtered_samples, sizeof(int16_t), num_samples, fp);
fclose(fp);
free(filtered_samples);
return 0;
}
```
这段代码实现了使用陷波法对wav文件进行啸叫抑制的功能。你可以根据自己的需求进行修改。
相关推荐
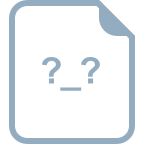














