import requests from bs4 import BeautifulSoup import pandas as pd # 获取网页数据 url = 'https://movie.douban.com/top250' response = requests.get(url) # 解析网页内容 soup = BeautifulSoup(response.text, 'html.parser') items = soup.find_all('div', class_='item') # 整理数据为 DataFrame data = [] for item in items: title = item.find('span', class_='title').text rating = item.find('span', class_='rating_num').text quote = item.find('span', class_='inq').text if item.find('span', class_='inq') else '' data.append([title, rating, quote]) df = pd.DataFrame(data, columns=['电影名称', '评分', '短评']) # 保存为 Excel 文件 df.to_excel('douban_top250.xlsx', index=False)我应该如何在python中运行呢
时间: 2024-01-10 22:02:24 浏览: 125
您可以在本地安装 Python 环境,并使用文本编辑器(如 VS Code、Sublime Text 等)打开一个新的 Python 文件,将上述代码复制粘贴到文件中。
然后在命令行中进入该 Python 文件所在的目录,输入以下命令来运行代码:
```
python 文件名.py
```
其中,`文件名.py` 是您保存的 Python 文件的文件名。如果您的 Python 环境配置正确,代码就会被执行,并且会在当前目录下生成一个名为 `douban_top250.xlsx` 的 Excel 文件。
相关问题
import requests from bs4 import BeautifulSoup import pandas as pd import matplotlib.pyplot as plt from PIL import Image import os # 定义请求头 headers = { 'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'} # 爬取页面的 URL url = 'https://movie.douban.com/top250' # 发送 GET 请求并获取响应 response = requests.get(url, headers=headers) html = response.text # 解析 HTML soup = BeautifulSoup(html, 'html.parser') # 获取电影列表 movies = [] for item in soup.find_all('div', class_='item'): movie = {} movie['rank'] = item.find('em').text movie['title'] = item.find('span', class_='title').text movie['director'] = item.find('div', class_='bd').p.text.split('\n')[1].strip().split(':')[1] movie['actors'] = item.find('div', class_='bd').p.text.split('\n')[2].strip()[3:] movie['score'] = item.find('span', class_='rating_num').text movie['votes'] = item.find('span', class_='rating_num').next_sibling.next_sibling.text[:-3] movie['cover_url'] = item.find('img')['src'] movies.append(movie) # 存储数据到 CSV 文件 df = pd.DataFrame(movies) df.to_csv('films.csv', index=False) # 下载封面图片并保存 if not os.path.exists('films_pic'): os.mkdir('films_pic') for movie in movies: img_url = movie['cover_url'] img_title = movie['title'] img_path = os.path.join('films_pic', f"{img_title}.jpg") response = requests.get(img_url, headers=headers) with open(img_path, 'wb') as f: f.write(response.content) # 可视化评分和人数 plt.plot(df['rank'], df['score'], label='Score') plt.plot(df['rank'], df['votes'], label='Votes') plt.xlabel('Rank') plt.legend() plt.savefig('score_votes.png') plt.show()
这段代码是一个爬取豆瓣电影 Top250 榜单信息的爬虫,主要实现以下功能:
1. 发送 GET 请求并获取响应,获取电影列表页面的 HTML 代码。
2. 解析 HTML 代码,获取电影信息,包括电影排名、电影名称、导演、演员、评分、评价人数、封面图片 URL。
3. 将电影信息存储到 DataFrame 中,并将 DataFrame 存储为 CSV 文件。
4. 下载电影封面图片,并保存到本地。
5. 可视化电影排名、评分、评价人数的关系,生成图表并保存到本地。
可以看出,这段代码主要使用了 requests 库、BeautifulSoup 库、pandas 库、matplotlib 库、os 库和 PIL 库。其中,requests 库用于发送 HTTP 请求,BeautifulSoup 库用于解析 HTML 代码,pandas 库用于处理数据,matplotlib 库用于绘制图表,os 库用于创建目录,PIL 库用于打开和保存图片。
需要注意的是,这段代码中的请求头 headers 可能不够隐蔽,存在被封禁的风险。建议在使用时根据实际情况调整请求头。此外,爬虫行为可能会违反豆瓣的相关规定,建议在合理使用的前提下进行爬取。
import requests from bs4 import BeautifulSoup url = 'https://movie.douban.com/top250' headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'} movies = [] for page in range(0, 250, 25): params = {'start': page, 'filter': ''} response = requests.get(url, headers=headers, params=params) soup = BeautifulSoup(response.text, 'html.parser') movie_list = soup.find('ol', class_='grid_view').find_all('li') for movie in movie_list: title = movie.find('span', class_='title').text rating = movie.find('span', class_='rating_num').text info = movie.find('div', class_='bd').p.text.strip().split('\n') director_actor = info[0] time_type = info[1].lstrip() movies.append((title, rating, director_actor, time_type)) for movie in movies: print(movie[0], movie[1], movie[2], movie[3])存储到execl中
要将数据存储到Excel中,你可以使用Python中的pandas库来实现。首先,你需要将数据转换为pandas的DataFrame格式,然后使用to_excel()函数将其保存为Excel文件。以下为示例代码:
```python
import pandas as pd
# 将数据转换为DataFrame格式
df = pd.DataFrame(movies, columns=['电影名称', '评分', '导演/演员', '时长/类型'])
# 保存为Excel文件
df.to_excel('top250_movies.xlsx', index=False)
```
这段代码将DataFrame命名为df,将movies中的数据列分别命名为电影名称、评分、导演/演员和时长/类型。然后,使用to_excel()函数将数据保存为top250_movies.xlsx文件,其中index=False表示不包含行索引。
阅读全文
相关推荐
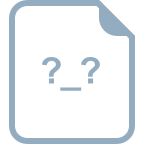
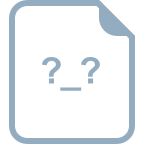
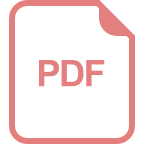




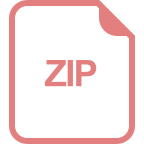









