如何在python实现LSTM的GridSearchCV参数寻优
时间: 2023-11-16 12:28:04 浏览: 219
在Python中,我们可以使用Scikit-learn库中的GridSearchCV函数来实现LSTM的参数寻优。具体步骤如下:
1. 首先,我们需要导入所需的库和数据。例如:
```python
import numpy as np
from keras.models import Sequential
from keras.layers import LSTM, Dense
from sklearn.model_selection import GridSearchCV
from sklearn.metrics import make_scorer, mean_squared_error
# 导入数据
X_train, y_train, X_test, y_test = load_data()
```
2. 接下来,我们需要定义LSTM模型。我们可以使用Sequential函数来定义模型,并添加LSTM和Dense层。例如:
```python
# 定义LSTM模型
def create_model(activation='relu', units=50, optimizer='adam'):
model = Sequential()
model.add(LSTM(units=units, activation=activation, input_shape=(X_train.shape[1], X_train.shape[2])))
model.add(Dense(1))
model.compile(loss='mean_squared_error', optimizer=optimizer)
return model
```
其中,activation、units和optimizer是需要进行参数寻优的参数。我们可以在create_model函数中设置默认值,并在GridSearchCV函数中进行参数搜索。
3. 然后,我们需要定义参数网格。我们可以使用Python中的字典来定义参数网格。例如:
```python
# 定义参数网格
param_grid = {'activation': ['relu', 'tanh', 'sigmoid'],
'units': [50, 100, 150],
'optimizer': ['adam', 'rmsprop']}
```
其中,activation、units和optimizer是需要进行参数搜索的参数。我们可以设置不同的参数值,并在GridSearchCV函数中进行搜索。
4. 接下来,我们需要使用GridSearchCV函数来搜索最佳参数。例如:
```python
# 定义评分函数
scorer = make_scorer(mean_squared_error, greater_is_better=False)
# 定义GridSearchCV函数
grid = GridSearchCV(estimator=create_model(), param_grid=param_grid, scoring=scorer, cv=3)
# 训练模型
grid.fit(X_train, y_train)
# 输出最佳参数
print(grid.best_params_)
```
其中,estimator参数是要优化的模型,param_grid是参数网格,scoring是评分函数,cv是交叉验证的折数。我们可以使用fit函数训练模型,并使用best_params_属性输出最佳参数。
希望这些步骤可以帮助你实现LSTM的GridSearchCV参数寻优。
相关推荐












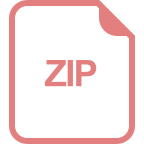
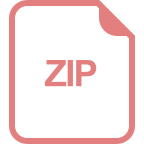
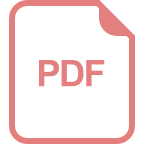