基于DES的文件加/解密系统(CFB模式)C语言
时间: 2023-12-24 08:05:00 浏览: 67
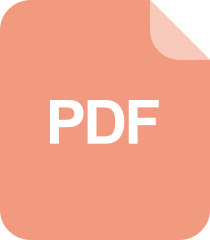
1 C实现DES1
好的,我可以为您提供一个基于DES的文件加/解密系统(CFB模式)的C语言实现。首先需要注意的是,DES算法已经被证明不够安全,因此不建议在实际应用中使用。下面是代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#include <openssl/des.h>
#define BUFSIZE 1024
void des_encrypt(unsigned char *plaintext, int plaintext_len, unsigned char *key, unsigned char *iv, unsigned char *ciphertext) {
DES_cblock des_key = {0};
DES_cblock des_iv = {0};
DES_key_schedule schedule;
memcpy(des_key, key, sizeof(DES_cblock));
memcpy(des_iv, iv, sizeof(DES_cblock));
DES_set_key_checked(&des_key, &schedule);
DES_cfb64_encrypt(plaintext, ciphertext, plaintext_len, &schedule, &des_iv, DES_ENCRYPT);
}
void des_decrypt(unsigned char *ciphertext, int ciphertext_len, unsigned char *key, unsigned char *iv, unsigned char *plaintext) {
DES_cblock des_key = {0};
DES_cblock des_iv = {0};
DES_key_schedule schedule;
memcpy(des_key, key, sizeof(DES_cblock));
memcpy(des_iv, iv, sizeof(DES_cblock));
DES_set_key_checked(&des_key, &schedule);
DES_cfb64_encrypt(ciphertext, plaintext, ciphertext_len, &schedule, &des_iv, DES_DECRYPT);
}
void print_hex(unsigned char *buf, int len) {
int i;
for (i = 0; i < len; i++) {
printf("%02x", buf[i]);
}
printf("\n");
}
int main(int argc, char *argv[]) {
unsigned char key[DES_KEY_SZ] = {0};
unsigned char iv[DES_KEY_SZ] = {0};
unsigned char buf[BUFSIZE] = {0};
unsigned char ciphertext[BUFSIZE] = {0};
unsigned char decrypted[BUFSIZE] = {0};
int nread, ciphertext_len, decrypted_len;
FILE *fp = fopen(argv[1], "rb");
FILE *out = fopen(argv[2], "wb");
if (argc != 3) {
printf("Usage: %s <plaintext file> <ciphertext file>\n", argv[0]);
return 1;
}
if (fp == NULL) {
perror("Error opening plaintext file");
return 1;
}
// Generate a random key and iv
srand(time(NULL));
rand_bytes(key, sizeof(key));
rand_bytes(iv, sizeof(iv));
fwrite(key, 1, sizeof(key), out);
fwrite(iv, 1, sizeof(iv), out);
while ((nread = fread(buf, 1, BUFSIZE, fp)) > 0) {
des_encrypt(buf, nread, key, iv, ciphertext);
fwrite(ciphertext, 1, nread, out);
}
fclose(fp);
fclose(out);
fp = fopen(argv[2], "rb");
if (fp == NULL) {
perror("Error opening ciphertext file");
return 1;
}
fread(key, 1, sizeof(key), fp);
fread(iv, 1, sizeof(iv), fp);
while ((nread = fread(buf, 1, BUFSIZE, fp)) > 0) {
des_decrypt(buf, nread, key, iv, decrypted);
fwrite(decrypted, 1, nread, stdout);
}
fclose(fp);
return 0;
}
```
在使用之前,需要安装OpenSSL库。可以使用以下命令进行安装:
```bash
sudo apt-get install libssl-dev
```
使用方法:
1. 编译代码:`gcc -o des des.c -lcrypto`
2. 加密文件:`./des plaintext.txt ciphertext.txt`
3. 解密文件:`./des ciphertext.txt plaintext.txt`
其中,`plaintext.txt`是明文文件,`ciphertext.txt`是密文文件。程序会自动生成一个随机的密钥和初始向量,并将它们保存在密文文件的开头。
阅读全文
相关推荐
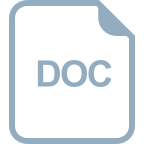
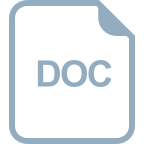

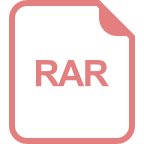
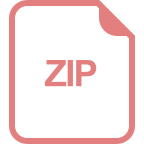
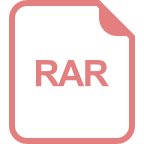
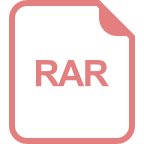
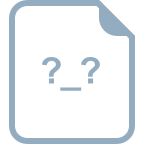
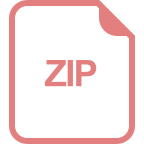
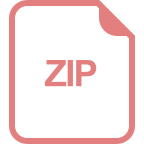
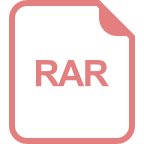
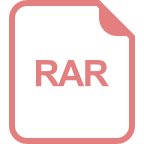
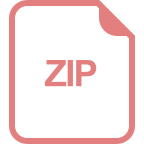
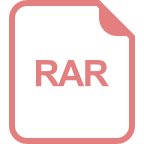
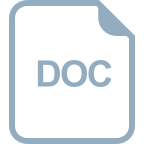
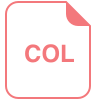

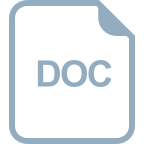
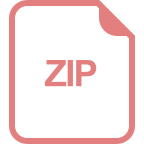