使用python语言基于面向对象思想利用paramiko远程进服务器,使用多线程批量ping并声音报警
时间: 2024-05-14 22:17:00 浏览: 22
您的问题是如何使用Python语言基于面向对象思想利用Paramiko远程进服务器,使用多线程批量ping并声音报警。
首先,您可以使用Paramiko库来建立一个SSH连接。下面是一些样例代码:
```python
import paramiko
import sys
class SSH:
def __init__(self, hostname, username, password):
self._ssh = paramiko.SSHClient()
self._ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
self._ssh.connect(hostname, username = username, password = password)
def execute(self, command):
stdin, stdout, stderr = self._ssh.exec_command(command)
if stderr.read():
print("Failed to run command: " + command)
return None
return stdout.readlines()
#测试
ssh = SSH('localhost', 'user', 'password')
output = ssh.execute("ls -l")
print(output)
```
其中,`SSH`类的构造函数通过`paramiko`库连接到指定主机,并提供用户名和密码。`execute`方法执行给定的命令并返回输出。测试代码展示了如何创建`SSH`对象并执行简单的命令。
接下来,您可以使用多线程来批量ping主机。下面是一些样例代码:
```python
import threading
import subprocess
import re
class Ping(threading.Thread):
def __init__(self, ip):
threading.Thread.__init__(self)
self.ip = ip
self.status = None
def run(self):
ping_output = subprocess.Popen(['ping', '-c', '1', self.ip], stdout = subprocess.PIPE).communicate()[0]
self.status = re.findall("100% packet loss", ping_output.decode('utf-8'))
#测试
threads = []
iplist = ['127.0.0.1', '127.0.0.2']
for ip in iplist:
thread = Ping(ip)
thread.start()
threads.append(thread)
for thread in threads:
thread.join()
if thread.status:
print(ip + "is down!")
else:
print(ip + "is up!")
```
以上简单地演示了如何使用多线程ping某些IP,并查看是否有失去任何数据包。如果某个IP无法到达,程序会输出警告。
最后,您可以使用Python库来播放声音。下面是一些参考代码:
```python
import pygame
class Sound:
def __init__(self, filename):
pygame.mixer.init()
pygame.mixer.music.load(filename)
def play(self):
pygame.mixer.music.play()
def stop(self):
pygame.mixer.music.stop()
#测试
sound = Sound('/path/to/your/sound/file.mp3')
sound.play()
```
在以上代码中,`Sound`类使用pygame库初始化音乐播放器。`play`方法播放指定的音频文件。测试代码展示了如何创建`Sound`对象并播放音频文件。
这样,您可以将上述三个组件组合在一起,以实现对大量主机的远程监控以及警报,即在向所有目标ping命令的同时,使用多线程执行该过程,并在服务器未响应时发送警报声音。
希望对您有所帮助!
相关推荐
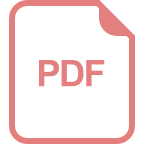
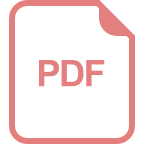
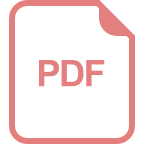














