根据输入的节点数和边数完成一个图,完成图的深度优先遍历算法、广度优先遍历算法。其中,邻接表的创建相当于创建多个单链表。用c++语言实现并给出注释
时间: 2024-03-10 11:46:13 浏览: 15
好的,以下是C++代码实现:
```cpp
#include <iostream>
#include <vector>
#include <queue>
using namespace std;
// 定义图的边结构体
struct Edge {
int to; // 边的终点
int weight; // 边的权重
Edge(int t, int w): to(t), weight(w) {}
};
// 定义图的邻接表结构体
struct Graph {
int n; // 节点数
vector<vector<Edge>> adj; // 邻接表
Graph(int n): n(n), adj(n) {}
// 添加一条边
void addEdge(int from, int to, int weight) {
adj[from].push_back(Edge(to, weight));
}
// 深度优先遍历
void dfs(int cur, vector<bool>& visited) {
visited[cur] = true;
cout << cur << " ";
for (auto e : adj[cur]) {
if (!visited[e.to]) {
dfs(e.to, visited);
}
}
}
// 广度优先遍历
void bfs(int cur, vector<bool>& visited) {
queue<int> q;
q.push(cur);
visited[cur] = true;
while (!q.empty()) {
int u = q.front();
q.pop();
cout << u << " ";
for (auto e : adj[u]) {
if (!visited[e.to]) {
visited[e.to] = true;
q.push(e.to);
}
}
}
}
};
int main() {
int n, m;
cin >> n >> m;
Graph g(n);
for (int i = 0; i < m; i++) {
int u, v, w;
cin >> u >> v >> w;
g.addEdge(u, v, w);
g.addEdge(v, u, w); // 无向图需要添加反向边
}
// 深度优先遍历
cout << "DFS: ";
vector<bool> visited(n, false);
g.dfs(0, visited);
cout << endl;
// 广度优先遍历
cout << "BFS: ";
visited.assign(n, false);
g.bfs(0, visited);
cout << endl;
return 0;
}
```
注释:
1. 定义了边结构体 `Edge` 和邻接表结构体 `Graph`,其中 `Edge` 包含了边的终点和权重,`Graph` 包含了节点数和邻接表数组。
2. `addEdge` 方法用于向邻接表中添加一条边,无向图需要添加反向边。
3. `dfs` 方法用于执行深度优先遍历,使用递归实现。`visited` 数组用于记录节点是否被访问过。
4. `bfs` 方法用于执行广度优先遍历,使用队列实现。`visited` 数组用于记录节点是否被访问过。
5. 在 `main` 函数中,首先读入节点数和边数,然后通过一个循环读入每条边的起点、终点和权重,最后执行深度优先遍历和广度优先遍历。
相关推荐
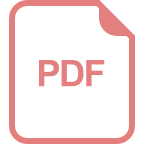
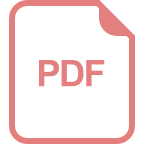
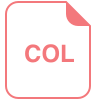
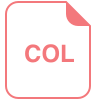
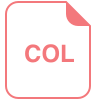
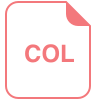
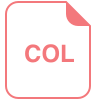









