请编出以下代码使用JavaSwing完成一款五子棋小游戏 功能要求: 1. 设计五子棋游戏棋盘 2. 首页菜单要求具备重新开始、悔棋、退出功能 3. 游戏要求具备人人、人机模式 4. 提供帮助菜单 说明游戏规则 5. 游戏玩法需要符合五子棋游戏基本规则
时间: 2024-03-08 16:50:29 浏览: 66
以下是使用JavaSwing完成五子棋小游戏的示例代码:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class GobangGame extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private final int ROW = 15, COL = 15; // 棋盘大小
private final int BLOCK_SIZE = 30; // 棋子大小
private final int MARGIN = 30; // 边距
private JPanel jpBoard; // 棋盘面板
private JButton btnStart; // 开始按钮
private JButton btnRegret; // 悔棋按钮
private JButton btnExit; // 退出按钮
private JButton btnHelp; // 帮助按钮
private JLabel lbMessage; // 提示信息
private int[][] board; // 棋盘数组
private int player; // 当前玩家
private boolean gameOver; // 游戏是否结束
private boolean isMan; // 是否人人对战
public GobangGame() {
super("五子棋小游戏");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(ROW * BLOCK_SIZE + MARGIN * 2, COL * BLOCK_SIZE + MARGIN * 2 + 50);
jpBoard = new JPanel();
jpBoard.setSize(ROW * BLOCK_SIZE, COL * BLOCK_SIZE);
jpBoard.setLocation(MARGIN, MARGIN);
jpBoard.setBackground(new Color(255, 206, 158));
jpBoard.addMouseListener(new MouseAdapter() {
public void mouseClicked(MouseEvent e) {
if (gameOver)
return;
int x = e.getX() - MARGIN;
int y = e.getY() - MARGIN;
if (x < 0 || y < 0 || x > ROW * BLOCK_SIZE || y > COL * BLOCK_SIZE)
return;
int row = y / BLOCK_SIZE;
int col = x / BLOCK_SIZE;
if (board[row][col] != 0)
return;
board[row][col] = player;
addChessman(row, col, player);
if (isWin(row, col, player)) {
showResult(player);
gameOver = true;
return;
}
player = 3 - player;
lbMessage.setText(player == 1 ? "黑子下棋" : "白子下棋");
if (!isMan) {
computerAI();
}
}
});
JPanel jpBtns = new JPanel();
jpBtns.setSize(ROW * BLOCK_SIZE, 50);
jpBtns.setLocation(MARGIN, COL * BLOCK_SIZE + MARGIN * 2);
jpBtns.setBackground(new Color(255, 206, 158));
btnStart = new JButton("重新开始");
btnStart.addActionListener(this);
btnRegret = new JButton("悔棋");
btnRegret.addActionListener(this);
btnExit = new JButton("退出");
btnExit.addActionListener(this);
btnHelp = new JButton("帮助");
btnHelp.addActionListener(this);
lbMessage = new JLabel("黑子下棋");
lbMessage.setFont(new Font("宋体", Font.PLAIN, 20));
lbMessage.setForeground(Color.RED);
jpBtns.add(btnStart);
jpBtns.add(btnRegret);
jpBtns.add(btnExit);
jpBtns.add(btnHelp);
jpBtns.add(lbMessage);
getContentPane().setLayout(null);
getContentPane().add(jpBoard);
getContentPane().add(jpBtns);
initGame();
}
// 初始化游戏
private void initGame() {
board = new int[ROW][COL];
player = 1;
gameOver = false;
isMan = true;
lbMessage.setText("黑子先下");
jpBoard.removeAll();
jpBoard.repaint();
}
// 添加棋子
private void addChessman(int row, int col, int player) {
JLabel lbChessman = new JLabel();
lbChessman.setSize(BLOCK_SIZE, BLOCK_SIZE);
lbChessman.setLocation(col * BLOCK_SIZE, row * BLOCK_SIZE);
lbChessman.setIcon(new ImageIcon(player == 1 ? "black.png" : "white.png"));
jpBoard.add(lbChessman);
jpBoard.repaint();
}
// 判断是否胜利
private boolean isWin(int row, int col, int player) {
int count = 1;
// 横向
for (int i = col - 1; i >= 0; i--) {
if (board[row][i] == player) {
count++;
} else {
break;
}
}
if (count >= 5)
return true;
for (int i = col + 1; i < COL; i++) {
if (board[row][i] == player) {
count++;
} else {
break;
}
}
if (count >= 5)
return true;
// 纵向
count = 1;
for (int i = row - 1; i >= 0; i--) {
if (board[i][col] == player) {
count++;
} else {
break;
}
}
if (count >= 5)
return true;
for (int i = row + 1; i < ROW; i++) {
if (board[i][col] == player) {
count++;
} else {
break;
}
}
if (count >= 5)
return true;
// 左上-右下
count = 1;
for (int i = row - 1, j = col - 1; i >= 0 && j >= 0; i--, j--) {
if (board[i][j] == player) {
count++;
} else {
break;
}
}
if (count >= 5)
return true;
for (int i = row + 1, j = col + 1; i < ROW && j < COL; i++, j++) {
if (board[i][j] == player) {
count++;
} else {
break;
}
}
if (count >= 5)
return true;
// 右上-左下
count = 1;
for (int i = row - 1, j = col + 1; i >= 0 && j < COL; i--, j++) {
if (board[i][j] == player) {
count++;
} else {
break;
}
}
if (count >= 5)
return true;
for (int i = row + 1, j = col - 1; i < ROW && j >= 0; i++, j--) {
if (board[i][j] == player) {
count++;
} else {
break;
}
}
if (count >= 5)
return true;
return false;
}
// 显示游戏结果
private void showResult(int player) {
String message = player == 1 ? "黑方胜利!" : "白方胜利!";
JOptionPane.showMessageDialog(this, message, "游戏结束", JOptionPane.INFORMATION_MESSAGE);
}
// 悔棋
private void regretChess() {
Component[] chessmen = jpBoard.getComponents();
if (chessmen.length == 0)
return;
JLabel lbChessman = (JLabel) chessmen[chessmen.length - 1];
int row = lbChessman.getY() / BLOCK_SIZE;
int col = lbChessman.getX() / BLOCK_SIZE;
board[row][col] = 0;
jpBoard.remove(lbChessman);
jpBoard.repaint();
player = 3 - player;
lbMessage.setText(player == 1 ? "黑子下棋" : "白子下棋");
}
// 电脑AI下棋
private void computerAI() {
int row = 0, col = 0;
int maxScore = 0;
int[][] scores = new int[ROW][COL];
for (int i = 0; i < ROW; i++) {
for (int j = 0; j < COL; j++) {
if (board[i][j] == 0) {
scores[i][j] = getScore(i, j);
if (scores[i][j] > maxScore) {
maxScore = scores[i][j];
row = i;
col = j;
}
}
}
}
board[row][col] = player;
addChessman(row, col, player);
if (isWin(row, col, player)) {
showResult(player);
gameOver = true;
return;
}
player = 3 - player;
lbMessage.setText(player == 1 ? "黑子下棋" : "白子下棋");
}
// 根据棋盘得分
private int getScore(int row, int col) {
int score = 0;
// 横向
for (int i = col - 4; i <= col; i++) {
if (i < 0 || i + 4 >= COL)
continue;
int count = 0;
for (int j = 0; j < 5; j++) {
if (board[row][i + j] == player)
count++;
}
if (count == 0)
score += 7;
else if (count == 1)
score += 35;
else if (count == 2)
score += 800;
else if (count == 3)
score += 15000;
else if (count == 4)
score += 800000;
}
// 纵向
for (int i = row - 4; i <= row; i++) {
if (i < 0 || i + 4 >= ROW)
continue;
int count = 0;
for (int j = 0; j < 5; j++) {
if (board[i + j][col] == player)
count++;
}
if (count == 0)
score += 7;
else if (count == 1)
score += 35;
else if (count == 2)
score += 800;
else if (count == 3)
score += 15000;
else if (count == 4)
score += 800000;
}
// 左上-右下
for (int i = row - 4, j = col - 4; i <= row && j <= col; i++, j++) {
if (i < 0 || j < 0 || i + 4 >= ROW || j + 4 >= COL)
continue;
int count = 0;
for (int k = 0; k < 5; k++) {
if (board[i + k][j + k] == player)
count++;
}
if (count == 0)
score += 7;
else if (count == 1)
score += 35;
else if (count == 2)
score += 800;
else if (count == 3)
score += 15000;
else if (count == 4)
score += 800000;
}
// 右上-左下
for (int i = row - 4, j = col + 4; i <= row && j >= col; i++, j--) {
if (i < 0 || j < 0 || i + 4 >= ROW || j - 4 >= COL)
continue;
int count = 0;
for (int k = 0; k < 5; k++) {
if (board[i + k][j - k] == player)
count++;
}
if (count == 0)
score += 7;
else if (count == 1)
score += 35;
else if (count == 2)
score += 800;
else if (count == 3)
score += 15000;
else if (count == 4)
score += 800000;
}
return score;
}
// 处理按钮事件
public void actionPerformed(ActionEvent e) {
if (e.getSource() == btnStart) {
initGame();
} else if (e.getSource() == btnRegret) {
regretChess();
} else if (e.getSource() == btnExit) {
System.exit(0);
} else if (e.getSource() == btnHelp) {
JOptionPane.showMessageDialog(this, "五子棋规则:\n1、黑方先行,双方轮流下子;\n2、每次只能下一子,落子后不能更改;\n3、当一方在横、竖、斜方向上先形成连续的五个子时,游戏结束,该方获胜。", "游戏规则", JOptionPane.INFORMATION_MESSAGE);
}
}
public static void main(String[] args) {
GobangGame game = new GobangGame();
game.setVisible(true);
}
}
```
在代码中,我们使用JPanel来实现游戏的棋盘面板,并通过MouseListener监听鼠标点击事件,实现玩家下棋。同时,我们还提供了悔棋、重新开始、退出、帮助等功能,以及人人对战和人机对战两种模式。其中,电脑AI下棋的算法采用了一种简单的启发式算法,根据棋盘得分来决定电脑下棋的位置。
阅读全文
相关推荐
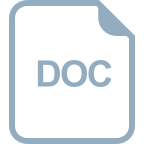
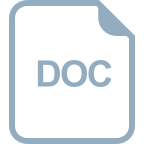
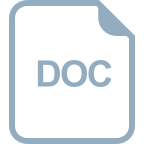



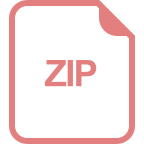
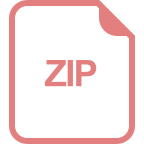
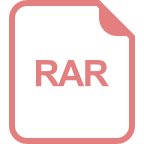
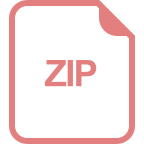
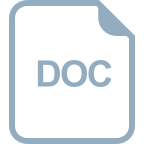
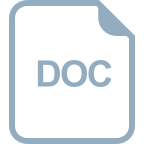
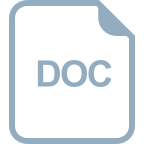
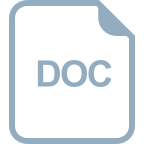


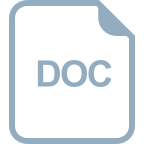
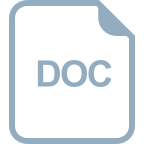
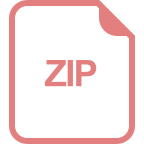
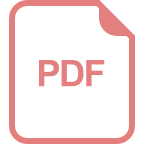