python实现DBN数据预测,输入输出数据均为三维
时间: 2024-03-02 07:53:15 浏览: 60
以下是使用Python实现DBN进行三维数据预测的示例代码:
```python
import numpy as np
import tensorflow as tf
from sklearn.model_selection import train_test_split
# 生成随机三维数据,输入为x,输出为y
x = np.random.rand(100, 10, 5)
y = np.random.rand(100, 1)
# 数据集分割
x_train, x_test, y_train, y_test = train_test_split(x, y, test_size=0.2, random_state=42)
# 定义网络参数
n_visible = 50
n_hidden_1 = 25
n_hidden_2 = 10
# 定义网络输入
x_input = tf.placeholder(tf.float32, [None, n_visible])
# 定义权重和偏置
W_1 = tf.Variable(tf.truncated_normal([n_visible, n_hidden_1], stddev=0.1))
b_1 = tf.Variable(tf.zeros([n_hidden_1]))
W_2 = tf.Variable(tf.truncated_normal([n_hidden_1, n_hidden_2], stddev=0.1))
b_2 = tf.Variable(tf.zeros([n_hidden_2]))
W_3 = tf.Variable(tf.zeros([n_hidden_2, 1]))
b_3 = tf.Variable(tf.zeros([1]))
# 定义网络模型
def network(input):
hidden_1 = tf.nn.sigmoid(tf.matmul(input, W_1) + b_1)
hidden_2 = tf.nn.sigmoid(tf.matmul(hidden_1, W_2) + b_2)
output = tf.matmul(hidden_2, W_3) + b_3
return output
# 定义损失函数和优化器
y_pred = network(x_input)
y_true = tf.placeholder(tf.float32, [None, 1])
loss = tf.reduce_mean(tf.square(y_true - y_pred))
train_step = tf.train.GradientDescentOptimizer(0.1).minimize(loss)
# 定义评估模型的准确率
mse = tf.reduce_mean(tf.square(y_true - y_pred))
# 训练模型
sess = tf.InteractiveSession()
tf.global_variables_initializer().run()
for i in range(1000):
_, train_loss = sess.run([train_step, loss], feed_dict={x_input: x_train.reshape(-1, n_visible), y_true: y_train.reshape(-1, 1)})
if i % 100 == 0:
test_loss = sess.run(loss, feed_dict={x_input: x_test.reshape(-1, n_visible), y_true: y_test.reshape(-1, 1)})
print("Epoch:", i, "Train loss:", train_loss, "Test loss:", test_loss)
# 评估模型
test_mse = sess.run(mse, feed_dict={x_input: x_test.reshape(-1, n_visible), y_true: y_test.reshape(-1, 1)})
print("Test MSE:", test_mse)
```
这个示例代码实现了一个两层的DBN,用于三维数据的预测。代码使用TensorFlow实现,将输入数据x展平为二维,定义网络结构、损失函数、优化器和评估指标,并进行训练和测试。在训练过程中,使用reshape()函数将输入数据x从三维转换为二维。最后,使用测试集评估模型的性能,计算MSE(均方误差)。
阅读全文
相关推荐
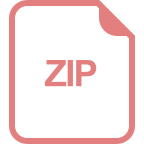
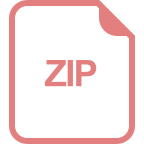
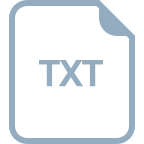
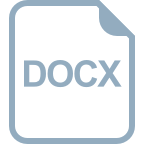
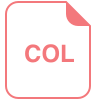
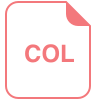
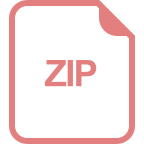
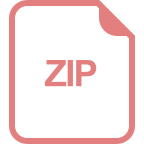