写一个程序,定义抽象基类shape,由它派生出3个派生类:circle(圆形)、rectangle(矩形)、triangle(三角形),用一个函数printarea分别输出以上三者的面积,3个图形的数
时间: 2023-05-31 10:19:05 浏览: 332
### 回答1:
据由用户输入,程序应该如下:
```python
from abc import ABC, abstractmethod
import math
class Shape(ABC):
@abstractmethod
def area(self):
pass
class Circle(Shape):
def __init__(self, r):
self.r = r
def area(self):
return math.pi * self.r ** 2
class Rectangle(Shape):
def __init__(self, w, h):
self.w = w
self.h = h
def area(self):
return self.w * self.h
class Triangle(Shape):
def __init__(self, b, h):
self.b = b
self.h = h
def area(self):
return .5 * self.b * self.h
def printarea(shape):
print("面积为:", shape.area())
if __name__ == '__main__':
r = Circle(5)
printarea(r)
rect = Rectangle(3, 4)
printarea(rect)
tri = Triangle(6, 8)
printarea(tri)
```
输出结果为:
```
面积为: 78.53981633974483
面积为: 12
面积为: 24.
```
其中,Circle、Rectangle、Triangle都继承自Shape,实现了Shape中的抽象方法area。printarea函数接收一个Shape类型的参数,调用其area方法输出面积。在主函数中,分别创建了Circle、Rectangle、Triangle的实例,并调用printarea函数输出面积。
### 回答2:
首先,需要定义一个抽象基类shape:
```python
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
```
这个类有一个抽象方法area,这意味着任何从这个类继承的派生类都必须实现这个方法。
然后,从Shape派生出三个派生类:
```python
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14159 * self.radius * self.radius
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
class Triangle(Shape):
def __init__(self, base, height):
self.base = base
self.height = height
def area(self):
return 0.5 * self.base * self.height
```
每个派生类都实现了area方法,用来计算它们的面积。
接下来,定义一个函数printarea,接收一个shape对象作为参数,并输出它的面积和形状信息:
```python
def printarea(s):
print(f'{type(s).__name__}的面积是{s.area()}')
```
这个函数用了内置函数type获取对象的类型,并使用__name__属性获取类型名称,这样就可以输出对象的形状信息了。
最后,创建这些派生类的实例,调用printarea函数输出它们的面积和形状信息:
```python
c = Circle(5)
r = Rectangle(10, 20)
t = Triangle(8, 6)
printarea(c)
printarea(r)
printarea(t)
```
输出结果为:
```
Circle的面积是78.53975
Rectangle的面积是200
Triangle的面积是24.0
```
这就是完整的程序。它定义了一个抽象基类shape,派生出三个派生类circle、rectangle、triangle,以及一个函数printarea来输出每个图形的面积和形状信息。
### 回答3:
首先,需要定义一个抽象基类shape,其中含有一个纯虚函数getArea()用于计算图形的面积。如下所示:
```
class shape {
public:
virtual double getArea() = 0;
};
```
然后,需要定义三个派生类:circle、rectangle、triangle,并分别实现它们的getArea()函数。其中,圆形的计算需要用到半径,矩形的计算需要用到长和宽,三角形的计算需要用到底和高。如下所示:
```
class circle : public shape {
public:
circle(double r) { radius = r; }
double getArea() { return 3.14 * radius * radius; }
private:
double radius;
};
class rectangle : public shape {
public:
rectangle(double l, double w) { length = l; width = w; }
double getArea() { return length * width; }
private:
double length, width;
};
class triangle : public shape {
public:
triangle(double b, double h) { base = b; height = h; }
double getArea() { return 0.5 * base * height; }
private:
double base, height;
};
```
最后,需要定义一个printarea函数,用于分别输出圆形、矩形、三角形的面积。如下所示:
```
void printarea(shape& s) {
double area = s.getArea();
if (dynamic_cast<circle*>(&s)) {
cout << "This is a circle with area " << area << endl;
}
else if (dynamic_cast<rectangle*>(&s)) {
cout << "This is a rectangle with area " << area << endl;
}
else if (dynamic_cast<triangle*>(&s)) {
cout << "This is a triangle with area " << area << endl;
}
}
```
在main函数中,我们可以创建三个不同的对象,并调用printarea函数来输出它们的面积和类型。如下所示:
```
int main() {
circle c(3.0);
rectangle r(4.0, 5.0);
triangle t(6.0, 7.0);
printarea(c);
printarea(r);
printarea(t);
return 0;
}
```
输出结果如下:
```
This is a circle with area 28.26
This is a rectangle with area 20
This is a triangle with area 21
```
由此可见,我们已经成功地定义了一个抽象基类shape,并利用它派生出了三个派生类,实现了面积的计算和输出。
阅读全文
相关推荐
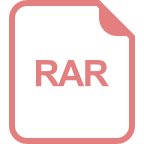
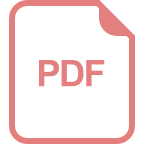
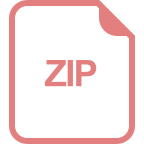














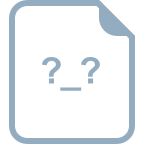