六子棋启发式搜索c语言代码
时间: 2023-07-30 10:12:56 浏览: 145
以下是六子棋启发式搜索的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define BOARD_SIZE 6
#define MAX_DEPTH 5
typedef struct {
int x;
int y;
} Point;
int board[BOARD_SIZE][BOARD_SIZE];
int player = 1;
int evaluate() {
int score = 0;
// 统计玩家和对手的连续棋子数,计算得分
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
if (board[i][j] == player) {
// 统计横向连续棋子数
int count = 1;
for (int k = 1; k < 6 && j + k < BOARD_SIZE; k++) {
if (board[i][j + k] == player) {
count++;
} else {
break;
}
}
if (count == 5) {
return 10000;
} else if (count > 1) {
score += count * count;
}
// 统计纵向连续棋子数
count = 1;
for (int k = 1; k < 6 && i + k < BOARD_SIZE; k++) {
if (board[i + k][j] == player) {
count++;
} else {
break;
}
}
if (count == 5) {
return 10000;
} else if (count > 1) {
score += count * count;
}
// 统计右上方向连续棋子数
count = 1;
for (int k = 1; k < 6 && i - k >= 0 && j + k < BOARD_SIZE; k++) {
if (board[i - k][j + k] == player) {
count++;
} else {
break;
}
}
if (count == 5) {
return 10000;
} else if (count > 1) {
score += count * count;
}
// 统计左上方向连续棋子数
count = 1;
for (int k = 1; k < 6 && i - k >= 0 && j - k >= 0; k++) {
if (board[i - k][j - k] == player) {
count++;
} else {
break;
}
}
if (count == 5) {
return 10000;
} else if (count > 1) {
score += count * count;
}
}
}
}
return score;
}
int alpha_beta_search(int depth, int alpha, int beta) {
if (depth == 0) {
return evaluate();
}
Point points[BOARD_SIZE * BOARD_SIZE];
int num_points = 0;
// 生成可行解
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
if (board[i][j] == 0) {
points[num_points].x = i;
points[num_points].y = j;
num_points++;
}
}
}
// 按照得分排序
for (int i = 0; i < num_points - 1; i++) {
for (int j = i + 1; j < num_points; j++) {
board[points[i].x][points[i].y] = player;
int score1 = evaluate();
board[points[i].x][points[i].y] = 0;
board[points[j].x][points[j].y] = player;
int score2 = evaluate();
board[points[j].x][points[j].y] = 0;
if (score2 > score1) {
Point temp = points[i];
points[i] = points[j];
points[j] = temp;
}
}
}
if (num_points == 0) {
return evaluate();
}
if (player == 1) {
int max_score = -10000;
for (int i = 0; i < num_points; i++) {
board[points[i].x][points[i].y] = player;
player = 2;
int score = alpha_beta_search(depth - 1, alpha, beta);
player = 1;
board[points[i].x][points[i].y] = 0;
if (score > max_score) {
max_score = score;
}
if (max_score >= beta) {
return max_score;
}
if (max_score > alpha) {
alpha = max_score;
}
}
return max_score;
} else {
int min_score = 10000;
for (int i = 0; i < num_points; i++) {
board[points[i].x][points[i].y] = player;
player = 1;
int score = alpha_beta_search(depth - 1, alpha, beta);
player = 2;
board[points[i].x][points[i].y] = 0;
if (score < min_score) {
min_score = score;
}
if (min_score <= alpha) {
return min_score;
}
if (min_score < beta) {
beta = min_score;
}
}
return min_score;
}
}
int main() {
// 初始化棋盘
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
board[i][j] = 0;
}
}
// 随机先手或后手
srand(time(NULL));
player = rand() % 2 + 1;
while (1) {
if (player == 1) {
// 玩家输入落子位置
int x, y;
printf("Your turn: ");
scanf("%d %d", &x, &y);
if (x < 0 || x >= BOARD_SIZE || y < 0 || y >= BOARD_SIZE || board[x][y] != 0) {
printf("Invalid move!\n");
continue;
}
board[x][y] = player;
// 判断玩家是否胜利
int score = evaluate();
if (score >= 10000) {
printf("You win!\n");
break;
}
player = 2;
} else {
// AI搜索最优解
int max_score = -10000;
Point best_point;
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
if (board[i][j] == 0) {
board[i][j] = player;
player = 2;
int score = alpha_beta_search(MAX_DEPTH, -10000, 10000);
player = 1;
board[i][j] = 0;
if (score > max_score) {
max_score = score;
best_point.x = i;
best_point.y = j;
}
}
}
}
printf("AI's turn: %d %d\n", best_point.x, best_point.y);
board[best_point.x][best_point.y] = player;
// 判断AI是否胜利
int score = evaluate();
if (score >= 10000) {
printf("AI wins!\n");
break;
}
player = 1;
}
}
return 0;
}
```
这段代码实现了基于α-β剪枝的六子棋启发式搜索算法,可以在控制台进行人机对弈。其中,evaluate()函数用于评估当前局面的得分,alpha_beta_search()函数用于执行α-β剪枝的搜索,main()函数实现了人机对弈的流程。
阅读全文
相关推荐
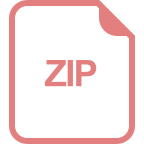
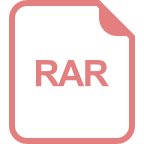
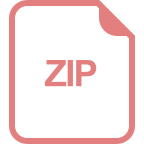















