python求字符串并集
时间: 2023-06-01 14:01:31 浏览: 109
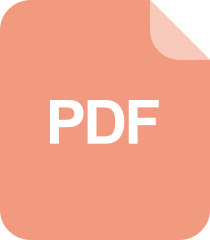
python字符串和常用数据结构知识总结
可以使用set()函数来求字符串的并集。
例如,假设有两个字符串s1和s2,要求它们的并集,可以如下操作:
```python
s1 = 'hello world'
s2 = 'world is beautiful'
# 将s1和s2转换为set类型
set1 = set(s1)
set2 = set(s2)
# 求并集
result_set = set1.union(set2)
# 将结果转换为字符串
result_str = ''.join(result_set)
print(result_str) # 输出结果为:d r o w e t i s b a f u l h l
```
上述代码中,首先将s1和s2转换为set类型,然后使用union()函数求它们的并集,最后将结果转换为字符串。
阅读全文
相关推荐
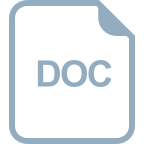
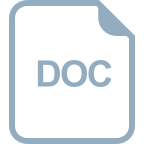


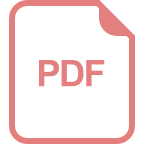







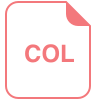




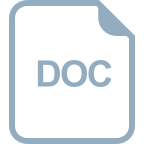