有一棵树,其结点的值为互不相同的正整数,这棵树用孩子兄弟表示法做存储结构,现输入一个正整数x。若树中存在值为x的结点,则输出该结点第2个孩子的值,若树中不存在值为x的结点,则输出:“no this node”,若树中值为x的结点没有孩子,或只有一个孩子,则输出:“no child of NO.2”。 输入描述 第一行是若干个正整数或“-1”组成的序列,是该树对应的二叉树(将该树转化成的二叉树)的扩展二叉树前序遍历序列,其中的“-1”表示空。第二行,一个正整数,表示x的值。 输出描述 树中值为x的结点第2个孩子的值。若树中不存在值为x的结点,则输出:“no this node”,若树中值为x的结点没有孩子,或只有一个孩子,则输出:“no child of NO.2”用java写
时间: 2023-06-14 12:04:48 浏览: 53
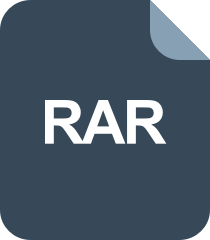
树的实现--利用二叉链表(孩子-兄弟)存储结构

```java
import java.util.*;
class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode(int x) { val = x; }
}
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String[] input = sc.nextLine().split(" ");
int x = sc.nextInt();
TreeNode root = buildTree(input);
TreeNode target = findNode(root, x);
if (target == null) {
System.out.println("no this node");
} else {
if (target.left == null || target.left.right == null) {
System.out.println("no child of NO.2");
} else {
System.out.println(target.left.right.val);
}
}
}
private static TreeNode buildTree(String[] input) {
Stack<TreeNode> stack = new Stack<>();
TreeNode root = new TreeNode(Integer.parseInt(input[0]));
stack.push(root);
for (int i = 1; i < input.length; i++) {
String s = input[i];
if (!s.equals("-1")) {
TreeNode node = new TreeNode(Integer.parseInt(s));
if (stack.peek().left == null) {
stack.peek().left = node;
} else {
stack.peek().right = node;
stack.pop();
}
stack.push(node);
} else {
stack.pop();
}
}
return root;
}
private static TreeNode findNode(TreeNode root, int x) {
if (root == null) {
return null;
}
if (root.val == x) {
return root;
}
TreeNode left = findNode(root.left, x);
if (left != null) {
return left;
}
TreeNode right = findNode(root.right, x);
if (right != null) {
return right;
}
return null;
}
}
```
阅读全文
相关推荐


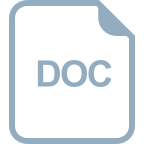
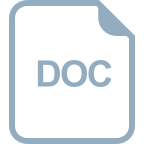
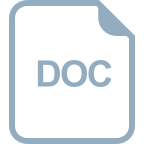
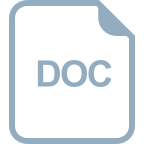
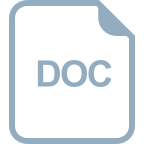
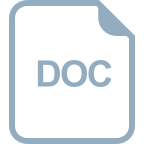
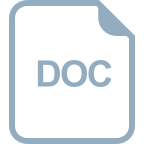
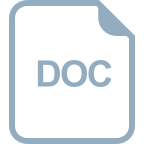
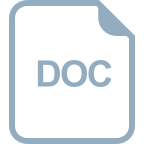
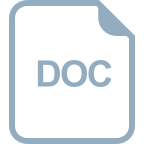
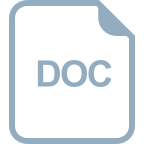
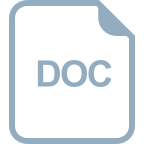
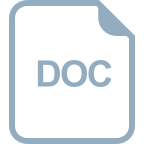