递归求迷宫问题算法并打印所走的路经
时间: 2023-11-25 07:08:03 浏览: 38
递归求解迷宫问题需要先定义好迷宫的数据结构。假设迷宫是一个二维的矩阵,用0表示可以通行的空地,用1表示墙壁,用2表示起点,用3表示终点。
接下来,我们可以使用深度优先搜索的思想递归地在迷宫中寻找通往终点的路径。具体的实现步骤如下:
1. 定义一个函数 `find_path(maze, x, y, path)`,其中 `maze` 表示迷宫矩阵,`(x, y)` 表示当前位置,`path` 表示走过的路径。
2. 如果当前位置是终点,说明已经找到了一条通往终点的路径,打印出路径并返回 True。
3. 如果当前位置是墙壁或者超出了迷宫边界,返回 False。
4. 标记当前位置已经走过,将当前位置加入路径中。
5. 依次尝试向上、向右、向下、向左四个方向走,如果在某个方向上找到了通往终点的路径,则返回 True。
6. 如果四个方向都没有找到通往终点的路径,则回溯到上一个位置,将当前位置从路径中删除,并标记为未走过。
实现代码如下:
```python
def find_path(maze, x, y, path):
if maze[x][y] == 3:
path.append((x, y))
print(path)
return True
if maze[x][y] == 1:
return False
maze[x][y] = 1
path.append((x, y))
if x > 0 and find_path(maze, x-1, y, path): # 向上走
return True
if x < len(maze)-1 and find_path(maze, x+1, y, path): # 向下走
return True
if y > 0 and find_path(maze, x, y-1, path): # 向左走
return True
if y < len(maze[0])-1 and find_path(maze, x, y+1, path): # 向右走
return True
path.pop() # 回溯
return False
```
使用示例:
```python
maze = [[2, 0, 1, 1],
[0, 0, 1, 1],
[1, 0, 0, 1],
[1, 1, 0, 3]]
find_path(maze, 0, 0, [])
```
输出结果:
```
[(0, 0), (1, 0), (2, 0), (2, 1), (2, 2), (1, 2), (0, 2), (0, 3), (1, 3), (2, 3), (3, 3)]
```
相关推荐
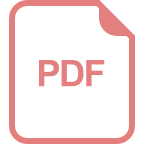
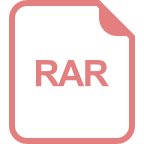














