mpu6050与stm32通信协议
时间: 2023-09-14 11:11:55 浏览: 36
MPU6050是一款集成了3轴陀螺仪、3轴加速度计和数字运动处理器的芯片,它通过I2C总线接口与MCU进行通信。通信协议如下:
1. I2C地址:MPU6050的I2C地址为0x68。
2. 寄存器地址:MPU6050内部有多个寄存器,通过寄存器地址来访问这些寄存器。
3. 读写操作:通过I2C总线进行读写操作,读取数据时需要先发送一个读取命令,然后再读取数据。
4. 数据格式:MPU6050返回的数据为16位的二进制补码形式,需要通过计算转换为实际值。
下面是一段基于HAL库的STM32与MPU6050通信的示例代码,可以供参考:
```c
#include "stm32f1xx_hal.h"
#define MPU6050_ADDR 0xD0
I2C_HandleTypeDef hi2c1;
void MPU6050_Init(void)
{
uint8_t data[2];
//使能MPU6050,设置采样率为1000Hz
data[0] = 0x6B;
data[1] = 0x00;
HAL_I2C_Mem_Write(&hi2c1, MPU6050_ADDR, 0x6B, 1, data, 1, 100);
//设置加速度计量程为±2g
data[0] = 0x1C;
data[1] = 0x00;
HAL_I2C_Mem_Write(&hi2c1, MPU6050_ADDR, 0x1C, 1, data, 1, 100);
//设置陀螺仪量程为±250dps
data[0] = 0x1B;
data[1] = 0x00;
HAL_I2C_Mem_Write(&hi2c1, MPU6050_ADDR, 0x1B, 1, data, 1, 100);
}
void MPU6050_Read_Acc(int16_t *acc)
{
uint8_t buf[6];
//读取加速度计XYZ轴的原始数据
HAL_I2C_Mem_Read(&hi2c1, MPU6050_ADDR, 0x3B, 1, buf, 6, 100);
//将读取到的数据转换为实际值
acc[0] = (int16_t)((buf[0] << 8) | buf[1]);
acc[1] = (int16_t)((buf[2] << 8) | buf[3]);
acc[2] = (int16_t)((buf[4] << 8) | buf[5]);
}
void MPU6050_Read_Gyro(int16_t *gyro)
{
uint8_t buf[6];
//读取陀螺仪XYZ轴的原始数据
HAL_I2C_Mem_Read(&hi2c1, MPU6050_ADDR, 0x43, 1, buf, 6, 100);
//将读取到的数据转换为实际值
gyro[0] = (int16_t)((buf[0] << 8) | buf[1]);
gyro[1] = (int16_t)((buf[2] << 8) | buf[3]);
gyro[2] = (int16_t)((buf[4] << 8) | buf[5]);
}
int main(void)
{
int16_t acc[3];
int16_t gyro[3];
HAL_Init();
__HAL_RCC_GPIOA_CLK_ENABLE();
__HAL_RCC_GPIOB_CLK_ENABLE();
__HAL_RCC_I2C1_CLK_ENABLE();
hi2c1.Instance = I2C1;
hi2c1.Init.ClockSpeed = 100000;
hi2c1.Init.DutyCycle = I2C_DUTYCYCLE_2;
hi2c1.Init.OwnAddress1 = 0;
hi2c1.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT;
hi2c1.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE;
hi2c1.Init.OwnAddress2 = 0;
hi2c1.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE;
hi2c1.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE;
HAL_I2C_Init(&hi2c1);
MPU6050_Init();
while (1)
{
MPU6050_Read_Acc(acc);
MPU6050_Read_Gyro(gyro);
//处理加速度计和陀螺仪的数据
//...
HAL_Delay(10);
}
}
```
相关推荐
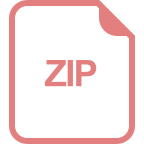
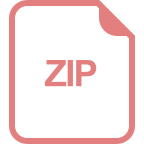














