def get_comments(url): headers = { 'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.36 Edge/16.16299' } response = requests.get(url, headers=headers) pattern = re.compile(r'"content":"(.*?)",', re.S) comments = pattern.findall(response.text) return comments 请告诉我这段爬虫代码具体是如何实现的
时间: 2024-04-21 15:29:51 浏览: 88
这段爬虫代码实现了获取指定 URL 中的评论内容,具体步骤如下:
1. 定义了一个函数 get_comments(),接收一个 URL 参数。
2. 设置了一个 headers 变量,用于模拟浏览器请求,避免被反爬机制拦截。
3. 通过 requests 库的 get() 方法向指定 URL 发送请求,并将响应结果赋值给 response 变量。
4. 使用正则表达式模块 re,定义了一个正则表达式模式 pattern,用于匹配评论内容。
5. 使用 re 模块的 findall() 方法在响应结果中查找所有匹配 pattern 的内容,并将结果赋值给 comments 变量。
6. 返回 comments 变量,即获取到的评论内容列表。
需要注意的是,这段代码仅适用于获取指定 URL 中通过 JSON 数据格式返回的评论内容,如果评论内容通过其他格式返回,需要根据实际情况修改代码。
相关问题
import requests def Jd_Spider(): # 传入url url = "https://api.m.jd.com/?appid=item-v3&functionId=pc_club_productPageComments&client=pc&clientVersion=1.0.0&t=1686224605129&loginType=3&uuid=122270672.16856900323181213839697.1685690032.1685690032.1686222590.2&productId=100052699188&score=0&sortType=5&page=0&pageSize=10&isShadowSku=0&fold=1&bbtf=&shield=" # 传入U-A headers = { 'user-agent': 'Mozilla/5.0 (Windows; U; Windows NT 6.1; en-US) AppleWebKit/534.17 (KHTML, like Gecko) Chrome/10.0.649.0 Safari/534.17' } # 传入ip地址 proxies = {'http': 'http://42.178.144.170:18394'} # get方法 resp = requests.get(url, headers=headers, proxies=proxies) resp.encoding = "utf-8" reso_json = resp.json() comment_list = reso_json['comments'] for comment in comment_list: # 商品id goods_id = comment.get('id') # 评论内容 content = comment.get('content') content = ' '.join(content.split('\n')) # 处理换行符 # 评分 score = comment.get('score') # 产品颜色 productColor = comment.get('productColor') # 产品尺寸 productSize = comment.get('productSize') # 地址 location = comment.get('location') # 引用名称 referenceName = comment.get('referenceName') print(f'{goods_id}\t{content}\t{score}\t{productColor}\t{productSize}\t{location}\t{referenceName}\n') pass if __name__ == '__main__': Jd_Spider()
这是一段 Python 代码,用于爬取京东商品的评论。代码中使用了 requests 库来发送 HTTP 请求,获取商品评论数据。其中,url 变量存储了需要爬取的京东商品评论 API 的地址,headers 变量存储了 HTTP 请求头,proxies 变量存储了代理服务器的地址。使用 requests 库的 get 方法向 API 发送请求,并将响应数据解析为 JSON 格式,然后从 JSON 数据中提取商品评论信息并输出。在 if __name__ == '__main__': 中调用 Jd_Spider 函数来执行代码。需要注意的是,使用爬虫程序爬取网站数据可能会违反网站的用户协议和法律法规,应该谨慎使用。
import requests from bs4 import BeautifulSoup from pprint import pprint import pymysql # 定义函数:将评论数据保存到数据库中 def save_to_navicat(comments): # 创建数据库连接 conn = pymysql.connect(host='localhost', user='root', password='root', db='pa', charset='utf8') # 创建游标对象 cursor = conn.cursor() # 插入数据到 MySQL 数据库中 for comment in comments: sql = ''' INSERT INTO comment (content, author, likes, time, location) VALUES (%s, %s, %s, %s, %s) ''' try: # 执行 SQL 插入语句 cursor.execute(sql, (comment['content'], comment['author'], comment['likes'], comment['time'], comment['location'])) # 提交事务 conn.commit() except Exception as e: # 如果发生异常,回滚事务 conn.rollback() print('Insert error:', e) # 关闭游标和连接 cursor.close() conn.close() for page in range(5): url = "https://movie.douban.com/subject/30391186/comments?start={}&limit=20&sort=new_score&status=P".format(page*20) headers = { "User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3" } response = requests.get(url, headers=headers) soup = BeautifulSoup(response.text, "html.parser") comments = [] for comment in soup.select(".comment-item"): content = comment.select_one(".comment-content").get_text().strip() author = comment.select_one(".comment-info a").get_text().strip() likes = comment.select_one(".votes").get_text().strip() time = comment.select_one(".comment-time").get_text().strip() location = comment.select_one(".comment-location").contents[-1].strip() comments.append({ "content": content, "author": author, "likes": likes, "time": time, "location": location }) print("第{}页的数据:".format(page+1)) pprint(comments),为什么数据库没有数据,完善代码
在您提供的代码中,缺少了将评论数据保存到数据库的步骤。您需要在获取到评论数据后,调用 `save_to_navicat()` 函数将数据保存到 MySQL 数据库中。您可以在代码中加入如下代码:
```
# 将获取到的评论数据保存到数据库中
save_to_navicat(comments)
```
这样,获取到的评论数据就会被保存到 MySQL 数据库中了。同时,需要注意数据库的配置是否正确,包括主机名、用户名、密码、数据库名等信息都需要正确配置。
阅读全文
相关推荐
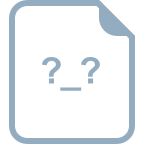
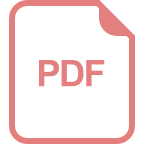
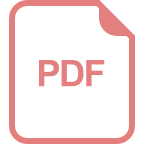
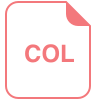
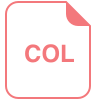
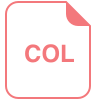
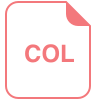
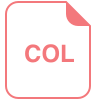








