stm32单片机如何控制四轮驱动麦克纳姆轮小车
时间: 2023-11-30 18:04:37 浏览: 357
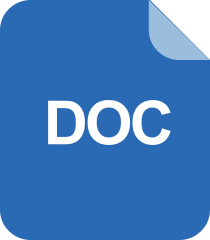
基于STM32的小车控制

控制四轮驱动麦克纳姆轮小车需要用到PWM信号控制电机的转速和方向,同时还需要使用编码器实时反馈电机的转速和位置,以便精确控制小车的运动。
以下是一个简单的控制程序示例,可以供参考:
1. 首先定义各个电机的控制引脚和编码器引脚:
```C
#define PWM_TIM TIM3
#define PWM_CH1 GPIO_Pin_6
#define PWM_CH2 GPIO_Pin_7
#define PWM_CH3 GPIO_Pin_8
#define PWM_CH4 GPIO_Pin_9
#define ENCODER_TIM TIM4
#define ENCODER_CH1 GPIO_Pin_6
#define ENCODER_CH2 GPIO_Pin_7
#define ENCODER_CH3 GPIO_Pin_8
#define ENCODER_CH4 GPIO_Pin_9
```
2. 初始化PWM信号和编码器:
```C
void PWM_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
TIM_TimeBaseInitTypeDef TIM_TimeBaseInitStruct;
TIM_OCInitTypeDef TIM_OCInitStruct;
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM3, ENABLE);
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOC, ENABLE);
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_100MHz;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_InitStructure.GPIO_Pin = PWM_CH1 | PWM_CH2 | PWM_CH3 | PWM_CH4;
GPIO_Init(GPIOC, &GPIO_InitStructure);
GPIO_PinAFConfig(GPIOC, GPIO_PinSource6, GPIO_AF_TIM3);
GPIO_PinAFConfig(GPIOC, GPIO_PinSource7, GPIO_AF_TIM3);
GPIO_PinAFConfig(GPIOC, GPIO_PinSource8, GPIO_AF_TIM3);
GPIO_PinAFConfig(GPIOC, GPIO_PinSource9, GPIO_AF_TIM3);
TIM_TimeBaseInitStruct.TIM_Prescaler = 0;
TIM_TimeBaseInitStruct.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseInitStruct.TIM_Period = 3999;
TIM_TimeBaseInitStruct.TIM_ClockDivision = TIM_CKD_DIV1;
TIM_TimeBaseInitStruct.TIM_RepetitionCounter = 0;
TIM_TimeBaseInit(PWM_TIM, &TIM_TimeBaseInitStruct);
TIM_OCInitStruct.TIM_OCMode = TIM_OCMode_PWM1;
TIM_OCInitStruct.TIM_OutputState = TIM_OutputState_Enable;
TIM_OCInitStruct.TIM_OCPolarity = TIM_OCPolarity_High;
TIM_OCInitStruct.TIM_Pulse = 0;
TIM_OC1Init(PWM_TIM, &TIM_OCInitStruct);
TIM_OC2Init(PWM_TIM, &TIM_OCInitStruct);
TIM_OC3Init(PWM_TIM, &TIM_OCInitStruct);
TIM_OC4Init(PWM_TIM, &TIM_OCInitStruct);
TIM_Cmd(PWM_TIM, ENABLE);
}
void Encoder_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
TIM_TimeBaseInitTypeDef TIM_TimeBaseInitStruct;
TIM_ICInitTypeDef TIM_ICInitStruct;
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM4, ENABLE);
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_100MHz;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_InitStructure.GPIO_Pin = ENCODER_CH1 | ENCODER_CH2 | ENCODER_CH3 | ENCODER_CH4;
GPIO_Init(GPIOB, &GPIO_InitStructure);
GPIO_PinAFConfig(GPIOB, GPIO_PinSource6, GPIO_AF_TIM4);
GPIO_PinAFConfig(GPIOB, GPIO_PinSource7, GPIO_AF_TIM4);
GPIO_PinAFConfig(GPIOB, GPIO_PinSource8, GPIO_AF_TIM4);
GPIO_PinAFConfig(GPIOB, GPIO_PinSource9, GPIO_AF_TIM4);
TIM_TimeBaseInitStruct.TIM_Prescaler = 0;
TIM_TimeBaseInitStruct.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseInitStruct.TIM_Period = 65535;
TIM_TimeBaseInitStruct.TIM_ClockDivision = TIM_CKD_DIV1;
TIM_TimeBaseInitStruct.TIM_RepetitionCounter = 0;
TIM_TimeBaseInit(ENCODER_TIM, &TIM_TimeBaseInitStruct);
TIM_ICInitStruct.TIM_Channel = TIM_Channel_1;
TIM_ICInitStruct.TIM_ICPolarity = TIM_ICPolarity_Rising;
TIM_ICInitStruct.TIM_ICSelection = TIM_ICSelection_DirectTI;
TIM_ICInitStruct.TIM_ICPrescaler = TIM_ICPSC_DIV1;
TIM_ICInitStruct.TIM_ICFilter = 0;
TIM_ICInit(ENCODER_TIM, &TIM_ICInitStruct);
TIM_ICInitStruct.TIM_Channel = TIM_Channel_2;
TIM_ICInitStruct.TIM_ICPolarity = TIM_ICPolarity_Rising;
TIM_ICInitStruct.TIM_ICSelection = TIM_ICSelection_DirectTI;
TIM_ICInitStruct.TIM_ICPrescaler = TIM_ICPSC_DIV1;
TIM_ICInitStruct.TIM_ICFilter = 0;
TIM_ICInit(ENCODER_TIM, &TIM_ICInitStruct);
TIM_ICInitStruct.TIM_Channel = TIM_Channel_3;
TIM_ICInitStruct.TIM_ICPolarity = TIM_ICPolarity_Rising;
TIM_ICInitStruct.TIM_ICSelection = TIM_ICSelection_DirectTI;
TIM_ICInitStruct.TIM_ICPrescaler = TIM_ICPSC_DIV1;
TIM_ICInitStruct.TIM_ICFilter = 0;
TIM_ICInit(ENCODER_TIM, &TIM_ICInitStruct);
TIM_ICInitStruct.TIM_Channel = TIM_Channel_4;
TIM_ICInitStruct.TIM_ICPolarity = TIM_ICPolarity_Rising;
TIM_ICInitStruct.TIM_ICSelection = TIM_ICSelection_DirectTI;
TIM_ICInitStruct.TIM_ICPrescaler = TIM_ICPSC_DIV1;
TIM_ICInitStruct.TIM_ICFilter = 0;
TIM_ICInit(ENCODER_TIM, &TIM_ICInitStruct);
TIM_EncoderInterfaceConfig(ENCODER_TIM, TIM_EncoderMode_TI12, TIM_ICPolarity_Rising, TIM_ICPolarity_Rising);
TIM_SetCounter(ENCODER_TIM, 0);
TIM_Cmd(ENCODER_TIM, ENABLE);
}
```
3. 编写PWM控制函数:
```C
void SetPWM(uint16_t ch1, uint16_t ch2, uint16_t ch3, uint16_t ch4)
{
TIM_SetCompare1(PWM_TIM, ch1);
TIM_SetCompare2(PWM_TIM, ch2);
TIM_SetCompare3(PWM_TIM, ch3);
TIM_SetCompare4(PWM_TIM, ch4);
}
```
4. 编写电机控制函数:
```C
void SetMotorSpeed(int16_t speed1, int16_t speed2, int16_t speed3, int16_t speed4)
{
uint16_t pwm1, pwm2, pwm3, pwm4;
pwm1 = abs(speed1);
pwm2 = abs(speed2);
pwm3 = abs(speed3);
pwm4 = abs(speed4);
if (speed1 >= 0)
{
GPIO_SetBits(GPIOC, GPIO_Pin_0);
GPIO_ResetBits(GPIOC, GPIO_Pin_1);
}
else
{
GPIO_ResetBits(GPIOC, GPIO_Pin_0);
GPIO_SetBits(GPIOC, GPIO_Pin_1);
}
if (speed2 >= 0)
{
GPIO_SetBits(GPIOC, GPIO_Pin_2);
GPIO_ResetBits(GPIOC, GPIO_Pin_3);
}
else
{
GPIO_ResetBits(GPIOC, GPIO_Pin_2);
GPIO_SetBits(GPIOC, GPIO_Pin_3);
}
if (speed3 >= 0)
{
GPIO_SetBits(GPIOC, GPIO_Pin_4);
GPIO_ResetBits(GPIOC, GPIO_Pin_5);
}
else
{
GPIO_ResetBits(GPIOC, GPIO_Pin_4);
GPIO_SetBits(GPIOC, GPIO_Pin_5);
}
if (speed4 >= 0)
{
GPIO_SetBits(GPIOC, GPIO_Pin_6);
GPIO_ResetBits(GPIOC, GPIO_Pin_7);
}
else
{
GPIO_ResetBits(GPIOC, GPIO_Pin_6);
GPIO_SetBits(GPIOC, GPIO_Pin_7);
}
SetPWM(pwm1, pwm2, pwm3, pwm4);
}
```
5. 编写控制程序,通过串口接收控制指令并控制小车运动:
```C
int main(void)
{
// 初始化各个引脚和模块
PWM_Init();
Encoder_Init();
USART_Init();
int16_t vx, vy, vw;
int16_t speed1, speed2, speed3, speed4;
while (1)
{
// 从串口接收控制指令
USART_Receive(&vx, &vy, &vw);
// 计算电机转速
speed1 = vx - vy - vw;
speed2 = vx + vy + vw;
speed3 = vx + vy - vw;
speed4 = vx - vy + vw;
// 控制电机
SetMotorSpeed(speed1, speed2, speed3, speed4);
}
}
```
以上是一个简单的控制程序示例,实际应用中还需要根据实际情况进行调整和完善。
阅读全文
相关推荐

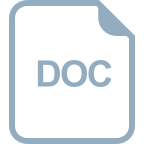
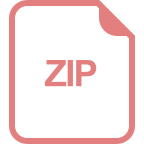
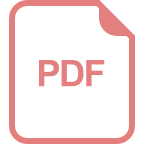
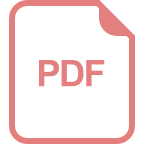
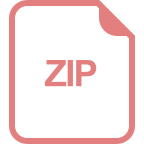
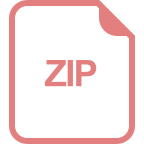
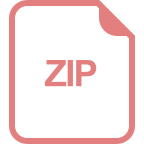
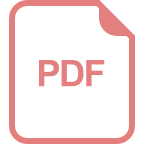
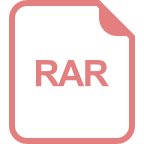
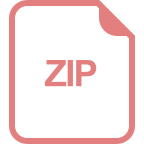
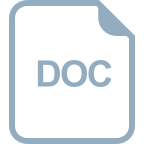
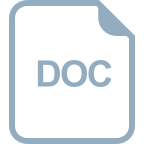
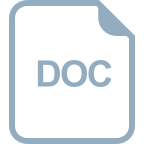
