以函数的形式实现监测/sys/class/gpio/gpio2是否有中断发生C语言
时间: 2023-07-07 08:34:13 浏览: 56
好的,以下是一个简单的以函数的形式实现监测 GPIO 引脚中断的 C 语言示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#include <string.h>
#include <poll.h>
#define GPIO_PIN_NUM 2
int check_gpio_interrupt(void) {
char gpio_path[50];
int gpio_fd;
struct pollfd gpio_poll;
char gpio_val[2];
// 构造 GPIO 引脚的路径
sprintf(gpio_path, "/sys/class/gpio/gpio%d/value", GPIO_PIN_NUM);
// 打开 GPIO 引脚的文件描述符
gpio_fd = open(gpio_path, O_RDONLY);
if (gpio_fd < 0) {
printf("Error: Failed to open GPIO pin!\n");
return -1;
}
// 配置 GPIO 引脚为输入模式
if (write("/sys/class/gpio/gpio2/direction", "in", 2) < 0) {
printf("Error: Failed to set GPIO direction!\n");
return -1;
}
// 监测 GPIO 引脚的中断
gpio_poll.fd = gpio_fd;
gpio_poll.events = POLLPRI | POLLERR;
if (poll(&gpio_poll, 1, -1) < 0) {
printf("Error: Failed to poll GPIO pin!\n");
return -1;
}
// 读取 GPIO 引脚的值
lseek(gpio_fd, 0, SEEK_SET);
read(gpio_fd, gpio_val, 2);
// 关闭 GPIO 引脚的文件描述符
close(gpio_fd);
// 处理 GPIO 引脚的中断
if (gpio_poll.revents & POLLPRI) {
printf("GPIO pin %d interrupted!\n", GPIO_PIN_NUM);
return 1;
}
return 0;
}
```
以上示例函数会打开指定 GPIO 引脚的文件描述符,并使用 `poll()` 函数来监测该引脚的中断状态。如果发生中断,函数会打印相应的信息并返回 1,否则返回 0。您可以在需要监测 GPIO 引脚中断的地方调用该函数。请注意,以上示例函数仅供参考,实际使用时可能需要根据具体情况进行修改和优化。
相关推荐
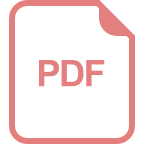
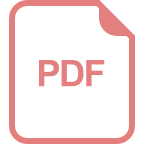
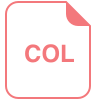













