C语言 Worksheets 读写excel表格实例
时间: 2024-01-19 17:04:34 浏览: 107
读写 Excel 表格是一项非常常见的操作,这里提供一个使用 C 语言读取和写入 Excel 表格的示例。
读取 Excel 表格:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
/* 引入第三方库libxls */
#include "libxls/xls.h"
int main(int argc, char *argv[]) {
xlsWorkBook *pWB;
xlsWorkSheet *pWS;
xlsCell *pCell = NULL;
int i, j;
/* 打开 Excel 文件 */
pWB = xls_open(argv[1], "UTF-8");
/* 获取第一个工作表 */
pWS = xls_getWorkSheet(pWB, 0);
/* 遍历单元格 */
for (i = pWS->rows.firstrow; i <= pWS->rows.lastrow; i++) {
for (j = pWS->cols.firstcol; j <= pWS->cols.lastcol; j++) {
pCell = xls_cell(pWS, i, j);
/* 打印单元格内容 */
if (pCell != NULL) {
printf("%s\t", pCell->str);
}
}
printf("\n");
}
/* 关闭 Excel 文件 */
xls_close(pWB);
return 0;
}
```
写入 Excel 表格:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
/* 引入第三方库libxls */
#include "libxls/xls.h"
int main(int argc, char *argv[]) {
xlsWorkBook *pWB;
xlsWorkSheet *pWS;
xlsCell *pCell = NULL;
int i, j;
/* 打开 Excel 文件 */
pWB = xls_open(argv[1], "UTF-8");
/* 获取第一个工作表 */
pWS = xls_getWorkSheet(pWB, 0);
/* 修改单元格内容 */
pCell = xls_cell(pWS, 1, 1);
if (pCell != NULL) {
strcpy(pCell->str, "New Value");
}
/* 保存 Excel 文件 */
xls_save(pWB, argv[1]);
/* 关闭 Excel 文件 */
xls_close(pWB);
return 0;
}
```
需要注意的是,这里使用了第三方库 `libxls` 来读取和写入 Excel 表格。在使用前需要先下载该库并进行编译。
阅读全文
相关推荐
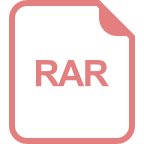
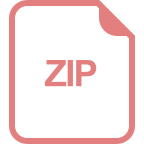
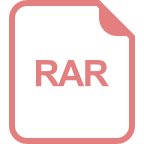
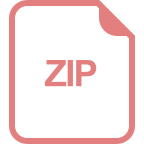




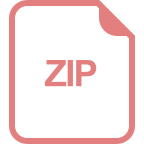
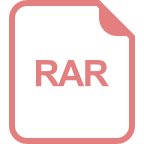
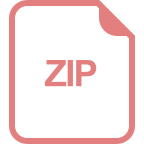
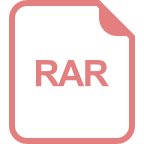
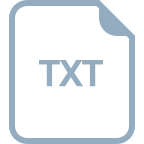
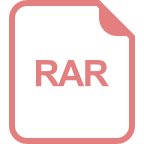
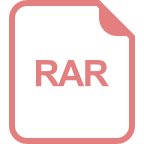
