C语言利用快速幂进行矩阵乘法运算
时间: 2023-09-27 18:11:30 浏览: 181
在C语言中,可以利用快速幂的思想实现矩阵乘法运算。具体实现步骤如下:
1. 定义一个矩阵结构体Matrix,包含行数rows、列数cols以及一个二维数组data表示矩阵中的元素。
```c
typedef struct {
int rows; // 矩阵的行数
int cols; // 矩阵的列数
int **data; // 矩阵的元素数组
} Matrix;
```
2. 实现矩阵乘法函数matrix_multiply,该函数输入两个矩阵A和B,并返回它们的乘积C。
```c
Matrix matrix_multiply(Matrix A, Matrix B) {
Matrix C;
C.rows = A.rows;
C.cols = B.cols;
C.data = (int **)malloc(C.rows * sizeof(int *));
for (int i = 0; i < C.rows; i++) {
C.data[i] = (int *)malloc(C.cols * sizeof(int));
memset(C.data[i], 0, C.cols * sizeof(int));
}
for (int i = 0; i < A.rows; i++) {
for (int k = 0; k < A.cols; k++) {
if (A.data[i][k] != 0) {
for (int j = 0; j < B.cols; j++) {
C.data[i][j] += A.data[i][k] * B.data[k][j];
}
}
}
}
return C;
}
```
3. 实现快速幂函数matrix_pow,该函数输入一个矩阵A和一个非负整数n,并返回A的n次幂。
```c
Matrix matrix_pow(Matrix A, int n) {
Matrix B;
B.rows = B.cols = A.rows;
B.data = (int **)malloc(B.rows * sizeof(int *));
for (int i = 0; i < B.rows; i++) {
B.data[i] = (int *)malloc(B.cols * sizeof(int));
memset(B.data[i], 0, B.cols * sizeof(int));
B.data[i][i] = 1; // 初始化为单位矩阵
}
while (n > 0) {
if (n % 2 == 1) {
B = matrix_multiply(B, A);
}
A = matrix_multiply(A, A);
n /= 2;
}
return B;
}
```
通过上述步骤,我们就可以利用快速幂实现矩阵乘法运算了。
阅读全文
相关推荐
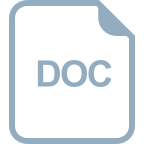
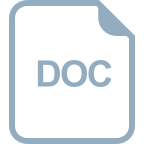
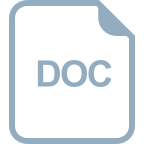


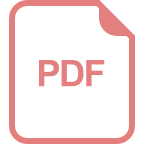
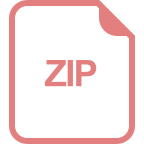
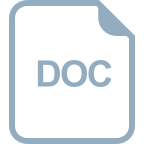
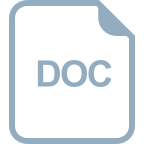
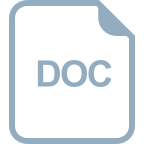
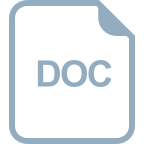
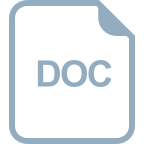
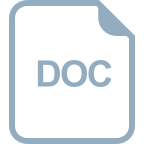
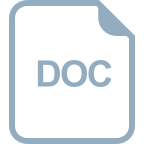
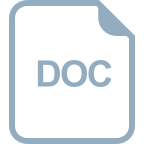
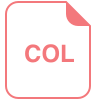

