使用c语言利用快速幂进行矩阵乘法运算
时间: 2023-09-26 17:13:45 浏览: 85
矩阵乘法可以使用快速幂算法优化,快速幂矩阵乘法可以在 $O(\log n)$ 的时间复杂度内计算矩阵的幂。下面是快速幂矩阵乘法的 C 语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define N 2 // 矩阵维度
// 定义矩阵结构体
struct Matrix {
int a[N][N];
};
// 初始化矩阵
void initMatrix(struct Matrix *m, int a11, int a12, int a21, int a22) {
m->a[0][0] = a11;
m->a[0][1] = a12;
m->a[1][0] = a21;
m->a[1][1] = a22;
}
// 矩阵乘法
struct Matrix matrixMultiply(struct Matrix m1, struct Matrix m2) {
struct Matrix res;
memset(&res, 0, sizeof(res));
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
for (int k = 0; k < N; k++) {
res.a[i][j] += m1.a[i][k] * m2.a[k][j];
}
}
}
return res;
}
// 快速幂矩阵乘法
struct Matrix matrixQuickPow(struct Matrix m, int n) {
struct Matrix res;
memset(&res, 0, sizeof(res));
for (int i = 0; i < N; i++) {
res.a[i][i] = 1; // 初始化为单位矩阵
}
while (n > 0) {
if (n & 1) {
res = matrixMultiply(res, m);
}
m = matrixMultiply(m, m);
n >>= 1;
}
return res;
}
int main() {
struct Matrix m;
initMatrix(&m, 1, 1, 1, 0); // 斐波那契数列的转移矩阵
int n;
scanf("%d", &n);
struct Matrix res = matrixQuickPow(m, n);
printf("斐波那契数列的第 %d 项为:%d\n", n, res.a[1][0]);
return 0;
}
```
在这个示例代码中,我们定义了一个矩阵结构体,包含矩阵的维度和元素,同时定义了初始化矩阵、矩阵乘法和快速幂矩阵乘法三个函数。在主函数中,我们输入矩阵的转移次数 $n$,然后使用快速幂矩阵乘法计算斐波那契数列的第 $n$ 项。
相关推荐
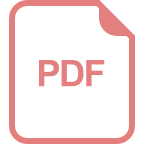
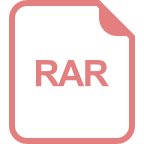
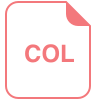
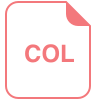












