python写一个类似matlab中的smooth五点三次平滑函数
时间: 2023-06-03 07:02:49 浏览: 57
好的,以下是Python实现五点三次平滑函数的代码:
```python
import numpy as np
def smooth(y):
"""
五点三次平滑函数
y: 一维数组
"""
N = len(y)
smoothed = np.zeros(N)
for i in range(2, N - 2):
smoothed[i] = (-3*y[i-2] + 12*y[i-1] + 17*y[i] + 12*y[i+1] - 3*y[i+2]) / 35
return smoothed
```
你可以将需要平滑的数据传递给函数 `smooth()`,并得到一个平滑处理后的数据数组。
相关问题
python写一个类似matlab中的smooth九点二次平滑函数
可以使用Python的Numpy库中的convolve函数实现九点二次平滑:
``` python
import numpy as np
def smooth(x, window_len=9, window='hanning'):
if window_len < 3:
return x
if window not in ['flat', 'hanning', 'hamming', 'bartlett', 'blackman']:
raise ValueError("Window is not one of 'flat', 'hanning', 'hamming', 'bartlett', 'blackman'")
s = np.r_[x[window_len-1:0:-1], x, x[-2:-window_len-1:-1]]
if window == 'flat': # moving average
w = np.ones(window_len, 'd')
else:
w = eval('np.' + window + '(window_len)')
y = np.convolve(w / w.sum(), s, mode='valid')
return y[(window_len // 2 - 1):-(window_len // 2)]
```
其中,x是输入数据,window_len指定平滑窗口大小(默认为9),window指定平滑窗口类型(默认为'hanning')。返回值y为平滑后的数据。
python写一个类似matlab中的smooth气象五点平滑函数
好的,这是一个关于 Python 编程的问题,我可以帮您解答。您可以使用 NumPy 库中的 convolve 函数,配合一个五点平均值的卷积核来实现。以下是一个示例代码:
```python
import numpy as np
def smooth(x, window_len=5, window='hanning'):
if window_len<3:
return x
if not window in ['flat', 'hanning', 'hamming', 'bartlett', 'blackman']:
raise ValueError("Window is not one of 'flat', 'hanning', 'hamming', 'bartlett', 'blackman'")
s=np.r_[x[window_len-1:0:-1], x, x[-2:-window_len-1: -1]]
if window == 'flat': # moving average
w=np.ones(window_len,'d')
else:
w=eval('np.'+window+'(window_len)')
y=np.convolve(w/w.sum(), s, mode='valid')
return y[(window_len/2-1):-(window_len/2)] #return smoothed signal
```
您可以通过传入一个一维数组 x,来获取平滑后的结果。其中,window_len 参数控制平滑窗口的大小,window 参数控制平滑的窗口类型。
相关推荐
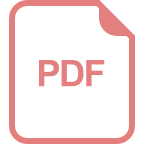
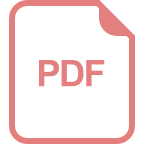












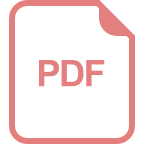