蚁群算法matlab代码栅格
时间: 2023-09-28 18:07:29 浏览: 92
以下是一个简单的蚁群算法在 Matlab 中的实现,用于解决栅格问题:
```matlab
function [best_path, best_dist] = ant_colony(grid, start, finish, num_ants, max_iter, alpha, beta, rho, q)
% grid: 栅格地图,1表示可行,0表示不可行
% start: 起点坐标
% finish: 终点坐标
% num_ants: 蚂蚁数量
% max_iter: 最大迭代次数
% alpha: 信息素重要程度
% beta: 启发式信息重要程度
% rho: 信息素挥发速度
% q: 信息素增加强度
% 初始化信息素浓度
pheromone = ones(size(grid));
% 计算启发式信息
heuristic = 1 ./ (grid + eps);
% 初始化最优路径和最短距离
best_path = [];
best_dist = Inf;
% 开始迭代
for iter = 1:max_iter
% 初始化蚂蚁的位置
curr_pos = repmat(start, num_ants, 1);
% 初始化蚂蚁的路径和距离
ant_path = zeros(num_ants, size(grid, 1) * size(grid, 2));
ant_dist = Inf(num_ants, 1);
% 开始移动蚂蚁
for step = 1:(size(grid, 1) * size(grid, 2) - 1)
% 计算蚂蚁在当前位置的可行移动方向
valid_moves = get_valid_moves(curr_pos, grid);
% 计算每个可行移动方向的转移概率
prob = get_prob(curr_pos, valid_moves, pheromone, heuristic, alpha, beta);
% 选择下一个位置
next_pos = choose_next_pos(valid_moves, prob);
% 更新路径和距离
ant_path(:, step) = sub2ind(size(grid), curr_pos(:, 1), curr_pos(:, 2));
ant_dist = ant_dist + sqrt(sum((curr_pos - next_pos).^2, 2));
% 更新当前位置并增加信息素
for i = 1:num_ants
pheromone(curr_pos(i, 1), curr_pos(i, 2)) = (1 - rho) * pheromone(curr_pos(i, 1), curr_pos(i, 2)) + q / ant_dist(i);
end
curr_pos = next_pos;
end
% 计算每只蚂蚁的路径是否为最优路径
for i = 1:num_ants
if ant_dist(i) < best_dist
best_dist = ant_dist(i);
best_path = ant_path(i, :);
end
end
% 更新信息素浓度
pheromone = (1 - rho) * pheromone;
for i = 1:length(best_path)-1
pheromone(best_path(i)) = pheromone(best_path(i)) + q / best_dist;
end
end
% 将路径转换为坐标
best_path = [mod(best_path-1, size(grid, 1))+1, ceil(best_path / size(grid, 1))];
end
function valid_moves = get_valid_moves(curr_pos, grid)
% 计算蚂蚁在当前位置的可行移动方向
valid_moves = [];
for dx = -1:1
for dy = -1:1
if dx == 0 && dy == 0
continue
end
pos = curr_pos + [dx, dy];
if all(pos >= 1) && all(pos <= size(grid)) && grid(pos(1), pos(2))
valid_moves = [valid_moves; pos];
end
end
end
end
function prob = get_prob(curr_pos, valid_moves, pheromone, heuristic, alpha, beta)
% 计算每个可行移动方向的转移概率
prob = zeros(size(valid_moves, 1), 1);
for i = 1:size(valid_moves, 1)
pos = valid_moves(i, :);
if any(curr_pos == pos)
prob(i) = 0;
else
prob(i) = pheromone(pos(1), pos(2))^alpha * heuristic(pos(1), pos(2))^beta;
end
end
prob = prob / sum(prob);
end
function next_pos = choose_next_pos(valid_moves, prob)
% 选择下一个位置
cum_prob = cumsum(prob);
r = rand;
next_pos = valid_moves(find(cum_prob >= r, 1), :);
end
```
在使用此代码时,需要先定义一个栅格地图 `grid`,其中 `1` 表示可行,`0` 表示不可行。然后定义起点 `start` 和终点 `finish` 的坐标。可以调整的参数有蚂蚁数量 `num_ants`、最大迭代次数 `max_iter`、信息素重要程度 `alpha`、启发式信息重要程度 `beta`、信息素挥发速度 `rho` 和信息素增加强度 `q`。运行此函数后,将会返回最优路径 `best_path` 和最短距离 `best_dist`。
阅读全文
相关推荐
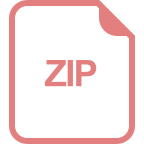
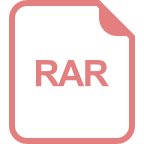
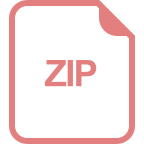
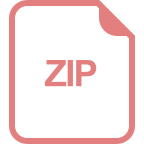
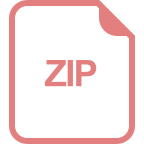
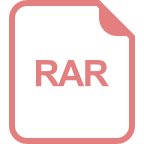
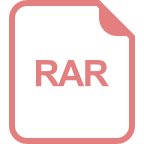



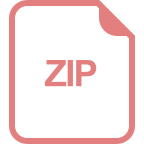
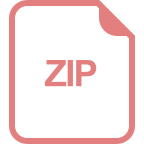


