opengl readfile函数
时间: 2023-10-18 20:19:05 浏览: 153
OpenGL本身并没有提供读取文件的函数,需要使用其他库来实现。常用的文件读取库有:
1. stdio.h:C语言标准库,提供了fopen()、fread()、fclose()等函数,可用于读取二进制文件。
2. fstream:C++标准库,提供了ifstream类,可用于读取文本文件。
3. SOIL(Simple OpenGL Image Library):开源的图像处理库,提供了SOIL_load_image()函数,可用于读取各种格式的图片文件。
4. Assimp(Open Asset Import Library):开源的模型导入库,可用于读取各种3D模型文件。
以下是一个使用fstream读取文本文件的例子:
```c++
#include <fstream>
#include <iostream>
using namespace std;
int main()
{
ifstream infile;
infile.open("test.txt"); // 打开文件
if (!infile)
{
cout << "文件打开失败" << endl;
return 0;
}
char c;
while (infile.get(c)) // 逐个字符读取文件内容
{
cout << c;
}
infile.close(); // 关闭文件
return 0;
}
```
相关问题
osg格式转opengl
osg格式是OpenSceneGraph的格式,用于描述三维场景。如果要将osg格式转换为OpenGL,则需要先将osg文件加载到内存中,然后解析其中的模型、材质、光照等信息,最终生成OpenGL需要的顶点数组、纹理数组、顶点索引等数据。
可以使用OpenSceneGraph库来加载osg文件,并使用其中的函数将其转换为OpenGL数据。具体步骤如下:
1. 安装OpenSceneGraph库,并将其包含到项目中。
2. 使用`osgDB::readNodeFile()`函数加载osg文件,并获取场景根节点。
3. 将场景根节点传递给`osgUtil::TriStripVisitor`类,该类将场景中的三角形面片转换为三角形条带。
4. 使用`osgUtil::TangentSpaceGenerator`类生成切线空间信息,用于在渲染时计算法线贴图。
5. 遍历场景节点,将每个节点的几何体转换为OpenGL顶点数组和纹理数组,并计算顶点索引。
6. 使用OpenGL函数将顶点数组、纹理数组、顶点索引等数据传递到GPU中。
7. 使用OpenGL函数进行渲染。
代码示例:
```c++
#include <osgDB/ReadFile>
#include <osgUtil/TriStripVisitor>
#include <osgUtil/TangentSpaceGenerator>
// 加载osg文件,返回场景根节点
osg::Node* loadOSGFile(const std::string& filename)
{
osg::ref_ptr<osg::Node> root = osgDB::readNodeFile(filename);
if (!root.valid())
{
std::cerr << "Failed to load file " << filename << std::endl;
return nullptr;
}
// 转换为三角形条带
osgUtil::TriStripVisitor tsv;
root->accept(tsv);
// 生成切线空间信息
osgUtil::TangentSpaceGenerator tsg;
tsg.generate(root);
return root.release();
}
// 将节点的几何体转换为OpenGL数据
void convertGeometry(osg::Geometry* geometry)
{
osg::Array* vertices = geometry->getVertexArray();
osg::Array* texcoords = geometry->getTexCoordArray(0);
osg::Vec3Array* normals = dynamic_cast<osg::Vec3Array*>(geometry->getNormalArray());
osg::DrawElements* indices = geometry->getDrawElements();
// TODO: 将vertices、texcoords、normals、indices转换为OpenGL数据
}
int main(int argc, char** argv)
{
// 加载osg文件
osg::Node* root = loadOSGFile("model.osg");
if (!root) return 1;
// 遍历场景节点,将几何体转换为OpenGL数据
osg::Geode* geode = root->asGeode();
for (unsigned int i = 0; i < geode->getNumDrawables(); ++i)
{
osg::Geometry* geometry = geode->getDrawable(i)->asGeometry();
convertGeometry(geometry);
}
// 渲染OpenGL数据
// TODO: 使用OpenGL函数进行渲染
return 0;
}
```
opengl绘制动画效果茶壶
要使用OpenGL绘制动画效果茶壶,可以按照以下步骤进行:
1. 加载茶壶模型
使用OpenGL的模型加载库,例如Assimp,加载茶壶模型。
2. 设置视图和投影矩阵
使用OpenGL的矩阵库,例如glm,设置视图和投影矩阵。
3. 设置光照
使用OpenGL的光照库,例如glLight,设置光照。
4. 绘制茶壶
使用OpenGL的绘制函数,例如glBegin和glEnd,绘制茶壶。
5. 实现动画效果
使用OpenGL的定时器,例如glutTimerFunc,实现动画效果。可以通过改变茶壶的位置,旋转角度或者大小来实现动画效果。
下面是一个使用OpenGL绘制动画效果茶壶的示例代码:
```c++
#include <GL/glut.h>
#include <glm/glm.hpp>
#include <glm/gtc/matrix_transform.hpp>
#include <glm/gtc/type_ptr.hpp>
#include <iostream>
#include <vector>
#include <assimp/Importer.hpp>
#include <assimp/scene.h>
#include <assimp/postprocess.h>
using namespace std;
// 定义茶壶模型
struct Model {
vector<GLfloat> vertices;
vector<GLfloat> normals;
};
Model teapotModel;
// 定义茶壶的位置和旋转角度
GLfloat teapotX = 0.0f;
GLfloat teapotY = 0.0f;
GLfloat teapotZ = -5.0f;
GLfloat teapotAngle = 0.0f;
// 加载茶壶模型
void loadTeapotModel() {
Assimp::Importer importer;
const aiScene* scene = importer.ReadFile("teapot.obj", aiProcess_Triangulate | aiProcess_GenSmoothNormals | aiProcess_FlipUVs);
if (!scene || scene->mFlags == AI_SCENE_FLAGS_INCOMPLETE || !scene->mRootNode) {
cout << "Error: Failed to load teapot model!" << endl;
return;
}
aiMesh* mesh = scene->mMeshes[0];
for (int i = 0; i < mesh->mNumFaces; i++) {
aiFace face = mesh->mFaces[i];
for (int j = 0; j < 3; j++) {
aiVector3D vertex = mesh->mVertices[face.mIndices[j]];
aiVector3D normal = mesh->mNormals[face.mIndices[j]];
teapotModel.vertices.push_back(vertex.x);
teapotModel.vertices.push_back(vertex.y);
teapotModel.vertices.push_back(vertex.z);
teapotModel.normals.push_back(normal.x);
teapotModel.normals.push_back(normal.y);
teapotModel.normals.push_back(normal.z);
}
}
}
// 初始化OpenGL
void init() {
glClearColor(0.0f, 0.0f, 0.0f, 1.0f);
glEnable(GL_DEPTH_TEST);
loadTeapotModel();
}
// 绘制茶壶
void drawTeapot() {
glEnableClientState(GL_VERTEX_ARRAY);
glEnableClientState(GL_NORMAL_ARRAY);
glVertexPointer(3, GL_FLOAT, 0, &teapotModel.vertices[0]);
glNormalPointer(GL_FLOAT, 0, &teapotModel.normals[0]);
glDrawArrays(GL_TRIANGLES, 0, teapotModel.vertices.size() / 3);
glDisableClientState(GL_VERTEX_ARRAY);
glDisableClientState(GL_NORMAL_ARRAY);
}
// 绘制场景
void display() {
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
glm::mat4 view = glm::lookAt(glm::vec3(0.0f, 0.0f, 5.0f), glm::vec3(0.0f, 0.0f, 0.0f), glm::vec3(0.0f, 1.0f, 0.0f));
glMultMatrixf(glm::value_ptr(view));
glm::mat4 projection = glm::perspective(glm::radians(45.0f), 1.0f, 0.1f, 100.0f);
glMultMatrixf(glm::value_ptr(projection));
glTranslatef(teapotX, teapotY, teapotZ);
glRotatef(teapotAngle, 0.0f, 1.0f, 0.0f);
drawTeapot();
glutSwapBuffers();
}
// 更新动画
void update(int value) {
teapotAngle += 1.0f;
if (teapotAngle > 360.0f) {
teapotAngle = 0.0f;
}
glutPostRedisplay();
glutTimerFunc(16, update, 0);
}
// 处理键盘事件
void keyboard(unsigned char key, int x, int y) {
switch (key) {
case 'w':
teapotY += 0.1f;
break;
case 's':
teapotY -= 0.1f;
break;
case 'a':
teapotX -= 0.1f;
break;
case 'd':
teapotX += 0.1f;
break;
case 'q':
teapotZ += 0.1f;
break;
case 'e':
teapotZ -= 0.1f;
break;
}
glutPostRedisplay();
}
// 主函数
int main(int argc, char* argv[]) {
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB | GLUT_DEPTH);
glutInitWindowSize(500, 500);
glutCreateWindow("OpenGL Teapot Animation");
init();
glutDisplayFunc(display);
glutTimerFunc(0, update, 0);
glutKeyboardFunc(keyboard);
glutMainLoop();
return 0;
}
```
在该示例代码中,我们使用了Assimp库加载茶壶模型,使用了glm库设置视图和投影矩阵,使用了glut库实现定时器和键盘事件处理。在display函数中,我们使用glTranslatef和glRotatef函数分别改变茶壶的位置和旋转角度。在update函数中,我们使用glutTimerFunc函数实现动画效果。在keyboard函数中,我们处理键盘事件,改变茶壶的位置。
阅读全文
相关推荐
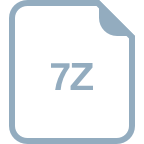
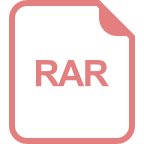
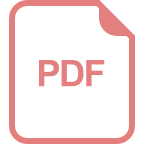
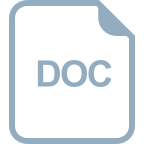
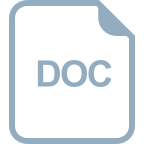




