c语言更改文件中的字符串
时间: 2023-06-23 14:50:28 浏览: 129
要更改文件中的字符串,您可以按照以下步骤进行:
1. 打开文件,并读取文件内容到内存中。
2. 在内存中查找要更改的字符串,并进行修改。
3. 将修改后的文件内容写回到原文件中。
下面是一个简单的示例程序,可以实现对文件中指定字符串的替换操作:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LINE_LENGTH 1024
int main(void)
{
char filename[] = "test.txt";
char old_str[] = "hello";
char new_str[] = "world";
char line[MAX_LINE_LENGTH];
FILE *fp_in, *fp_out;
// 打开文件
fp_in = fopen(filename, "r");
fp_out = fopen("temp.txt", "w");
// 逐行读取文件内容
while (fgets(line, MAX_LINE_LENGTH, fp_in) != NULL) {
// 在当前行查找要替换的字符串
char *pos = strstr(line, old_str);
while (pos != NULL) {
// 执行替换操作
memcpy(pos, new_str, strlen(new_str));
pos = strstr(pos + strlen(new_str), old_str);
}
// 写回修改后的行
fputs(line, fp_out);
}
// 关闭文件
fclose(fp_in);
fclose(fp_out);
// 删除原文件并将修改后的文件重命名为原文件名
remove(filename);
rename("temp.txt", filename);
printf("Done!\n");
return 0;
}
```
在这个示例程序中,我们将要修改的文件名、要替换的字符串、替换成的字符串都作为字符数组定义。在程序中,我们先打开原文件并创建一个临时文件。然后逐行读取原文件中的内容,通过 `strstr` 函数查找要替换的字符串,并使用 `memcpy` 函数执行替换操作。然后将修改后的行写入临时文件中。最后,我们删除原文件并将临时文件重命名为原文件名,即实现了对文件中指定字符串的替换操作。
阅读全文
相关推荐
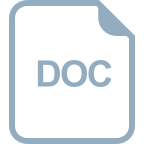
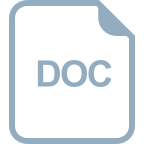
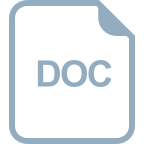

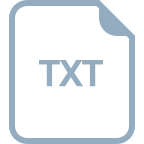
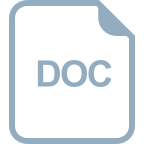
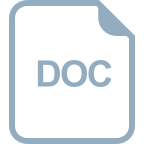
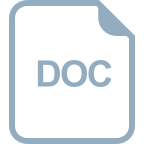

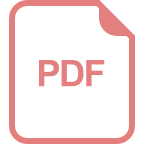
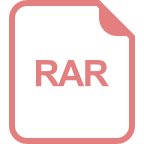
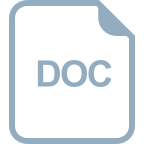
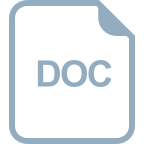
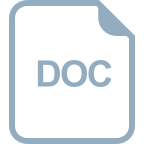
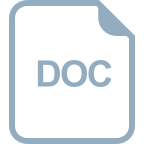
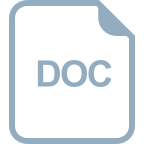
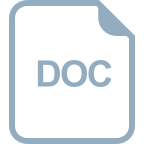
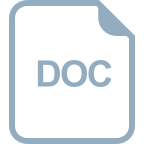
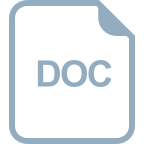