java实现仿射变换加解密算法(附完整源码)
时间: 2023-12-06 19:23:30 浏览: 194
仿射变换加密算法是一种基于线性代数的加密算法,它可以对明文进行加密并生成密文,同时可以对密文进行解密还原成明文。下面是一个 Java 实现仿射变换加解密算法的示例代码:
```java
public class AffineCipher {
private static final String ALPHABET = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
// 加密函数
public static String encrypt(String plainText, int a, int b) {
plainText = plainText.toUpperCase();
String cipherText = "";
for (int i = 0; i < plainText.length(); i++) {
char c = plainText.charAt(i);
if (c == ' ') {
cipherText += " ";
continue;
}
int x = ALPHABET.indexOf(c);
int y = (a * x + b) % 26;
cipherText += ALPHABET.charAt(y);
}
return cipherText;
}
// 解密函数
public static String decrypt(String cipherText, int a, int b) {
cipherText = cipherText.toUpperCase();
String plainText = "";
int aInverse = getInverse(a);
for (int i = 0; i < cipherText.length(); i++) {
char c = cipherText.charAt(i);
if (c == ' ') {
plainText += " ";
continue;
}
int y = ALPHABET.indexOf(c);
int x = (aInverse * (y - b + 26)) % 26;
plainText += ALPHABET.charAt(x);
}
return plainText;
}
// 获取 a 在模 26 意义下的乘法逆元
private static int getInverse(int a) {
for (int x = 1; x < 26; x++) {
if ((a * x) % 26 == 1) {
return x;
}
}
return -1;
}
public static void main(String[] args) {
String plainText = "HELLO WORLD";
int a = 3;
int b = 2;
String cipherText = encrypt(plainText, a, b);
System.out.println("Cipher text: " + cipherText);
String decryptedText = decrypt(cipherText, a, b);
System.out.println("Decrypted text: " + decryptedText);
}
}
```
在这个示例中,我们定义了一个 `AffineCipher` 类来实现仿射变换加密和解密。我们使用了英文字母表作为我们加密的字符集,即 `ALPHABET = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"`。
我们实现了两个函数来加密和解密字符串,分别为 `encrypt` 和 `decrypt`。加密函数接收明文、变换参数 `a` 和 `b`,并返回密文。解密函数接收密文、变换参数 `a` 和 `b`,并返回明文。
在加密和解密函数中,我们先将输入的字符串转换为大写字母,然后遍历字符串中的每个字符。对于空格字符,我们直接将其添加到输出字符串中,不进行加密或解密。对于其他字符,我们先将其转换为在字母表中的下标 `x`,然后使用仿射变换公式 $y = (ax + b) \bmod 26$ 进行加密或解密,并将结果转换为对应的字符。
我们还实现了一个 `getInverse` 函数来获取在模 26 意义下的 `a` 的乘法逆元。我们遍历 1 到 25 中的每个数 `x`,检查是否存在一个数使得 $ax \equiv 1 \pmod{26}$,如果找到则返回该数,否则返回 -1。
在主函数中,我们定义了一个明文 `HELLO WORLD`,并使用变换参数 `a = 3` 和 `b = 2` 进行加密。我们输出了加密后的密文,并使用相同的变换参数进行解密,输出了解密后的明文。
需要注意的是,仿射变换加密算法并不是一种安全的加密算法,它可以被比较容易地破解。在实际应用中,应该使用更加复杂的加密算法来保护数据的安全。
阅读全文
相关推荐







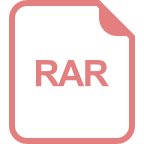


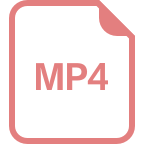



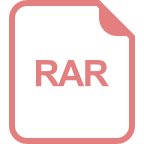