data = importdata('1-5.txt', ' '); x = data(:, 1); image = data(:, 2); % 获取波峰位置数据 [pks, locs] = findpeaks(image); % 归一化处理波峰位置数据 normalized_locs = normalize(locs, 'range'); % 自适应背景扣除 window_size = 30; % 窗口大小 corrected_image = zeros(size(image)); for i = 1:length(image) % 确定当前窗口的起始和结束位置 window_start = max(i - floor(window_size/2), 1); window_end = min(i + floor(window_size/2), length(image)); % 获取当前窗口内的背景估计 background = median(image(window_start:window_end)); % 扣除背景估计 corrected_image(i) = image(i) - background; end
时间: 2023-06-24 14:04:30 浏览: 146
这段代码的作用是对一个图像的波峰位置数据进行处理,包括归一化处理、自适应背景扣除等。
首先,通过`importdata`函数读取名为`1-5.txt`的文件,并将第一列数据存储到变量`x`中,将第二列数据存储到变量`image`中。
接下来,使用`findpeaks`函数寻找`image`中的波峰位置,并将波峰位置数据存储在`pks`和`locs`两个变量中。
然后,使用`normalize`函数对`locs`进行归一化处理,得到`normalized_locs`。
最后,使用一个`for`循环,对`image`中每一个像素点进行自适应背景扣除。具体来说,对于每一个像素点,确定当前窗口的起始和结束位置,计算窗口内的背景估计,并将背景估计值从该像素点的值中扣除,得到经过背景扣除后的值,存储在`corrected_image`中。其中,窗口大小由`window_size`指定,这里是取窗口大小的一半作为窗口半径,即`floor(window_size/2)`。
相关问题
import json import base64 from PIL import Image import io import cv2 from ultralytics import YOLO import supervision as sv def init_context(context): context.logger.info("Init context... 0%") model_path = "yolov8m-seg.pt" # YOLOV8模型放在nuclio目录下构建 model = YOLO(model_path) # Read the DL model context.user_data.model = model context.logger.info("Init context...100%") def handler(context, event): context.logger.info("Run yolo-v8-seg model") data = event.body buf = io.BytesIO(base64.b64decode(data["image"])) threshold = float(data.get("threshold", 0.35)) context.user_data.model.conf = threshold image = Image.open(buf) yolo_results = context.user_data.model(image, conf=threshold)[0] labels = yolo_results.names detections = sv.Detections.from_yolov8(yolo_results) detections = detections[detections.confidence > threshold] masks = detections.xy conf = detections.confidence class_ids = detections.class_id results = [] if masks.shape[0] > 0: for label, score, mask in zip(class_ids, conf, masks): # 将mask转换为轮廓 contours, _ = cv2.findContours(mask.astype(np.uint8), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE) for contour in contours: points = [] for point in contour: x = point[0][0] y = point[0][1] points.append([x, y]) results.append({ "confidence": str(score), "label": labels.get(label, "unknown"), "points": points, "type": "polygon", }) return context.Response(body=json.dumps(results), headers={}, content_type='application/json', status_code=200)还缺哪些包和库
根据你提供的代码,缺少以下库和包:
1. `numpy`:用于处理数组和矩阵操作。你可以使用`pip install numpy`安装它。
2. `ultralytics`:用于YOLO模型的推理和结果处理。你可以使用`pip install ultralytics`安装它。
确保在你的环境中安装了这些库,并在代码中进行导入:
```python
import numpy as np
from ultralytics import YOLO
```
请注意,这只是你代码中缺少的一些库和包,你可能还需要根据你的需求进行进一步的导入。如果还有其他缺失的库或包,请提供相关信息。
import json import base64 from PIL import Image import io import cv2 import numpy as np from ultralytics import YOLO import supervision as sv def init_context(context): context.logger.info("Init context... 0%") model_path = "yolov8m-seg.pt" # YOLOV8模型放在nuclio目录下构建 model = YOLO(model_path) # Read the DL model context.user_data.model = model context.logger.info("Init context...100%") def handler(context, event): context.logger.info("Run yolo-v8-seg model") data = event.body buf = io.BytesIO(base64.b64decode(data["image"])) threshold = float(data.get("threshold", 0.35)) context.user_data.model.conf = threshold image = Image.open(buf) yolo_results = context.user_data.model(image, conf=threshold)[0] labels = yolo_results.names detections = sv.Detections.from_yolov8(yolo_results) detections = detections[detections.confidence > threshold] masks = detections.xy conf = detections.confidence class_ids = detections.class_id results = [] if masks.shape[0] > 0: for label, score, mask in zip(class_ids, conf, masks): # 将mask转换为轮廓 contours, _ = cv2.findContours(mask.astype(np.uint8), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE) for contour in contours: points = [] for point in contour: x = point[0][0] y = point[0][1] points.append([x, y]) results.append({ "confidence": str(score), "label": labels.get(label, "unknown"), "points": points, "type": "polygon",}) return context.Response(body=json.dumps(results), headers={}, content_type='application/json', status_code=200)不用supervision 包 用别的方式解析
如果你不想使用 `supervision` 包,可以使用其他方式解析 `masks`。
以下是修改后的代码示例,使用 OpenCV 库中的 `cv2.findContours()` 函数和 `cv2.drawContours 函数来解析 `masks` 并结果列表:
```python
import json
import base64
from PIL import Image
import io
import cv2
import numpy as np
from ultralytics import YOLO
def init_context(context):
context.logger.info("Init context... 0%")
model_path = "yolov8m-seg.pt" # YOLOV8模型放在nuclio目录下构建
model = YOLO(model_path)
# Read the DL model
context.user_data.model = model
context.logger.info("Init context...100%")
def handler(context, event):
context.logger.info("Run yolo-v8-seg model")
data = event.body
buf = io.BytesIO(base64.b64decode(data["image"]))
threshold = float(data.get("threshold", 0.35))
context.user_data.model.conf = threshold
image = Image.open(buf)
yolo_results = context.user_data.model(image, conf=threshold)[0]
labels = yolo_results.names
detections = yolo_results.pred
results = []
for detection in detections:
class_id = int(detection[5])
score = detection[4]
mask = detection[6]
# 将mask转换为轮廓
_, contours, _ = cv2.findContours(mask.astype(np.uint8), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
for contour in contours:
points = []
for point in contour:
x = point[0][0]
y = point[0][1]
points.append([x, y])
results.append({
"confidence": str(score),
"label": labels[class_id],
"points": points,
"type": "polygon"
})
return context.Response(body=json.dumps(results), headers={},
content_type='application/json', status_code=200)
```
在这个修改后的代码中,我假设你已经正确导入了所需的库和模块,并且使用了 `YOLO` 类来获取预测结果。
我修改了 `handler` 函数中的代码,将 `detections` 对象中的每个检测结果进行遍历。对于每个检测结果,我提取了类别ID、置信度和掩码。然后,使用 `cv2.findContours()` 函数将掩码转换为轮廓,并使用 `cv2.drawContours()` 函数绘制轮廓。
最后,我将每个检测结果的类别、置信度和轮廓点添加到结果列表中。
请注意,这只是一个示例代码,可能需要根据你的实际需求进行调整和优化。
希望这能帮助到你!如果还有其他问题,请随时提问。
阅读全文
相关推荐
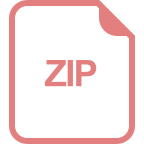
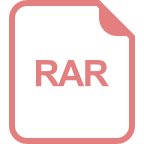
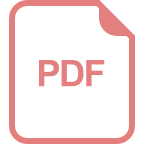














