def findClosestCentroids(X, centroids): #定义函数findClosestCentroids """ Returns the closest centroids in idx for a dataset X where each row is a single example. """ K = centroids.shape[0] #获得数组centroids的行数并赋值给K idx = np.zeros((X.shape[0],1)) #定义idx为X.shape[0]行1列的零数组 temp = np.zeros((centroids.shape[0],1)) #定义temp为centroids.shape[0]行1列的数组 for i in range(X.shape[0]): #i遍历循环X.shape[0] for j in range(K): #j遍历循环K dist = X[i,:] - centroids[j,:] # length = np.sum(dist**2) temp[j] = length idx[i] = np.argmin(temp)+1 return idx 给这段代码注释
时间: 2023-06-17 12:06:00 浏览: 118
# 定义函数findClosestCentroids,它接受两个参数:数据集X和聚类中心centroids
# 函数的作用是为数据集中的每个样本找到距离它最近的聚类中心,并将其对应的聚类中心下标存储在idx中
# 获取聚类中心的数量K
K = centroids.shape[0]
# 初始化idx为X.shape[0]行1列的零数组
idx = np.zeros((X.shape[0],1))
# 初始化temp为centroids.shape[0]行1列的数组
temp = np.zeros((centroids.shape[0],1))
# 遍历数据集X中的每个样本
for i in range(X.shape[0]):
# 遍历每个聚类中心
for j in range(K):
# 计算当前样本到聚类中心的距离
dist = X[i,:] - centroids[j,:]
# 将距离的平方和存储在temp数组中
length = np.sum(dist**2)
temp[j] = length
# 找到距离当前样本最近的聚类中心下标,并将其加1存储在idx中
idx[i] = np.argmin(temp)+1
# 返回存储聚类中心下标的idx
return idx
相关问题
def findClosestCentroids(X, centroids):
该函数是 K-Means 算法中的一个子函数,用于找到数据点 X 中每个点最近的聚类中心。具体来说,它输入数据矩阵 X 和聚类中心矩阵 centroids,输出一个向量 idx,其中 idx(i) 表示第 i 个数据点所属的最近的聚类中心的索引。
函数代码可能类似于下面这样:
```
import numpy as np
def findClosestCentroids(X, centroids):
K = centroids.shape[0] # 获取聚类中心的个数
m = X.shape[0] # 获取数据点的个数
idx = np.zeros(m) # 初始化 idx 向量
for i in range(m):
# 计算 X(i) 与每个聚类中心的距离
distances = np.sqrt(np.sum((X[i] - centroids) ** 2, axis=1))
# 找到距离最近的聚类中心的索引
idx[i] = np.argmin(distances)
return idx
```
其中,np.argmin(distances) 返回 distances 中最小值的索引。
idx = findClosestCentroids(X, centroids)
这是一个聚类算法中的函数,用于将数据集 X 中的每个样本点分配到离它最近的聚类中心点。具体来说,它接受两个参数:数据集 X 和聚类中心点 centroids,并返回一个长度为 m 的向量 idx,其中第 i 个元素是表示数据集中第 i 个样本点应该分配到哪个聚类中心点的下标,也就是离它最近的聚类中心点的下标。这个函数的实现方式一般有两种:暴力枚举和使用 k-d 树。暴力枚举的时间复杂度为 O(m * k),其中 m 是样本点数目,k 是聚类中心点数目;使用 k-d 树的时间复杂度为 O(m * log(k)),但需要先构建 k-d 树,构建的时间复杂度为 O(k * m * log(m))。
阅读全文
相关推荐
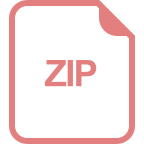
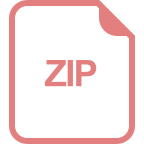
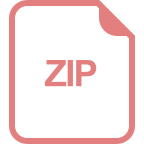













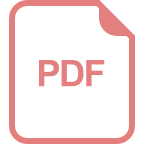