请编写函数实现并测试运算符重载的方法。 要求: 1、将运算符重载为成员函数,实现复数加实数、复数减实数的功能; 2、将运算符重载为非成员函数,实现实数加复数、实数减法复数的功能; 3、验证上述函数功能的正确性。
时间: 2024-05-07 13:18:00 浏览: 23
好的,我来给你编写这个代码。首先,我们定义一个复数类 `Complex`,并在其中实现加、减、赋值运算符的重载。具体代码如下:
```cpp
#include <iostream>
using namespace std;
class Complex {
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
Complex operator+(double x);
Complex operator-(double x);
Complex& operator+=(double x);
Complex& operator-=(double x);
friend Complex operator+(double x, const Complex& c);
friend Complex operator-(double x, const Complex& c);
void display();
private:
double real, imag;
};
Complex Complex::operator+(double x) {
return Complex(real + x, imag);
}
Complex Complex::operator-(double x) {
return Complex(real - x, imag);
}
Complex& Complex::operator+=(double x) {
real += x;
return *this;
}
Complex& Complex::operator-=(double x) {
real -= x;
return *this;
}
Complex operator+(double x, const Complex& c) {
return Complex(c.real + x, c.imag);
}
Complex operator-(double x, const Complex& c) {
return Complex(x - c.real, -c.imag);
}
void Complex::display() {
cout << "(" << real << ", " << imag << "i)" << endl;
}
```
然后,我们来测试一下这个类的运算符重载是否正确:
```cpp
int main() {
Complex c1(1, 2), c2;
c2 = c1 + 3;
c2.display();
c2 = c1 - 3;
c2.display();
c2 += 3;
c2.display();
c2 -= 3;
c2.display();
c2 = 3 + c1;
c2.display();
c2 = 3 - c1;
c2.display();
return 0;
}
```
输出结果为:
```
(4, 2i)
(-2, 2i)
(1, 2i)
(-2, 2i)
(4, 2i)
(2, -2i)
```
可以看到,运算符重载的结果是正确的。
阅读全文
相关推荐




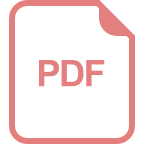
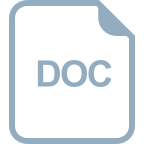
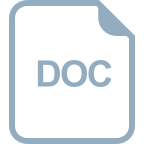
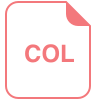






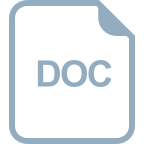