优化这段代码 function doDefaultValueFunction() { if (!getFunByKey.value) { return } const funList = globalJS.getListenerFun(getFunByKey.value) let errorMsg: string = '' if (funList.length > 0) { for (let fun of funList) { let msg = fun() errorMsg = errorMsg ? errorMsg : msg } } if (errorMsg) { showMessage({ type: 'warning', content: errorMsg }) } }
时间: 2024-03-01 11:50:04 浏览: 69
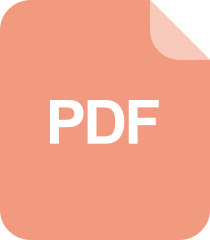
vuex 解决报错this.$store.commit is not a function的方法
可以优化的地方有:
1. 函数名不够明确,可以更改为更能描述其功能的名称。
2. 如果`getFunByKey.value`不存在,函数会直接返回,可以添加一个默认返回值,使函数更加健壮。
3. 在`for`循环中使用了`let`声明变量,这样可以避免变量提升的问题,建议继续使用。
4. 在循环中使用了三目运算符,不够简洁,可以改为使用逻辑或运算符`||`。
综合以上建议,可以将代码优化为:
```
function checkDefaultValue() {
if (!getFunByKey.value) {
return false;
}
const funList = globalJS.getListenerFun(getFunByKey.value);
let errorMsg = '';
for (let fun of funList) {
let msg = fun();
errorMsg = errorMsg || msg;
}
if (errorMsg) {
showMessage({
type: 'warning',
content: errorMsg
});
return false;
}
return true;
}
```
在以上代码中,函数名更加明确,添加了默认返回值`false`,优化了三目运算符,使代码更加简洁易读。
阅读全文
相关推荐
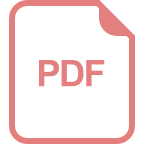
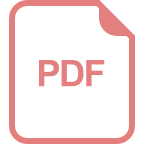













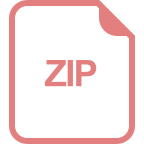
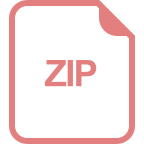
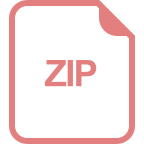
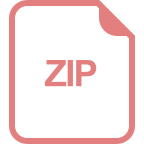