pthread_create如何正确使用
时间: 2023-09-02 07:14:03 浏览: 37
pthread_create 函数用于创建一个新的线程,并将其运行在指定的函数中。正确使用 pthread_create 函数需要注意以下几点:
1. 函数原型
```
int pthread_create(pthread_t *thread, const pthread_attr_t *attr, void *(*start_routine)(void*), void *arg);
```
参数说明:
* thread:指向 pthread_t 类型变量的指针,用于存储新线程的 ID。
* attr:指向 pthread_attr_t 类型变量的指针,用于设置新线程的属性,通常传入 NULL 表示使用默认属性。
* start_routine:指向新线程运行的函数的指针。
* arg:传递给新线程的参数,可以为任意类型的指针。
2. 函数返回值
pthread_create 函数返回值为 0 表示创建线程成功,否则表示创建线程失败,返回的错误代码可以通过 strerror 函数转换成错误信息。
3. 线程函数
新线程运行的函数需要满足以下要求:
* 函数返回类型为 void*,并接收一个 void* 类型的参数。
* 函数体内需要使用 pthread_exit 函数来结束线程。
4. 线程属性
如果需要设置线程属性,可以使用 pthread_attr_init 函数初始化 pthread_attr_t 类型的变量,然后使用 pthread_attr_setxxx 函数设置相应的属性,最后将变量传入 pthread_create 函数中。若不需要设置属性,则可以直接传入 NULL。
下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <pthread.h>
void *thread_func(void *arg) {
int *p = (int *)arg;
printf("new thread: %d\n", *p);
pthread_exit(NULL);
}
int main() {
pthread_t tid;
int arg = 100;
int ret = pthread_create(&tid, NULL, thread_func, &arg);
if (ret != 0) {
printf("create thread failed: %s\n", strerror(ret));
return 1;
}
printf("main thread: %d\n", arg);
pthread_exit(NULL);
}
```
在这个示例中,创建了一个新线程,并将参数 100 传递给线程函数 thread_func。线程函数打印出参数的值,然后使用 pthread_exit 函数结束线程。主线程也打印出参数的值,并使用 pthread_exit 函数结束。注意线程函数的返回值类型必须为 void*,但是在本示例中没有使用返回值,因此可以直接传入 NULL。
相关推荐
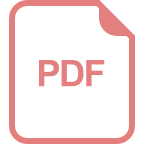
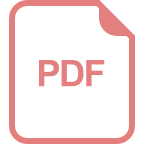
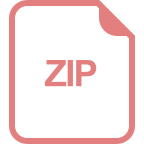














