uniapp 节流函数
时间: 2023-12-08 07:06:26 浏览: 54
在uniapp中可以使用以下代码实现一个简单的节流函数:
```
/**
* 节流函数
* @param {Function} fn 需要节流的函数
* @param {Number} delay 节流时间间隔
*/
function throttle(fn, delay) {
let lastTime = 0;
return function() {
const now = new Date().getTime();
if (now - lastTime > delay) {
fn.apply(this, arguments);
lastTime = now;
}
};
}
```
使用方法如下:
```
const handleScroll = throttle(function() {
// 需要进行节流的代码
}, 1000);
// 监听滚动事件
document.addEventListener('scroll', handleScroll);
```
在上面的例子中,`handleScroll` 函数会在每次滚动事件触发时被调用,但是由于加入了节流函数,实际上 `handleScroll` 函数会在每隔 1 秒钟才会被调用一次,从而避免了频繁触发函数的问题。
相关问题
uniapp 封装的防抖节流函数
以下是一个基于 Uniapp 框架封装的防抖节流函数:
```javascript
export function debounce(fn, delay = 500) {
let timer = null;
return function(...args) {
if (timer) clearTimeout(timer);
timer = setTimeout(() => {
fn.apply(this, args);
}, delay);
};
}
export function throttle(fn, interval = 500) {
let timer = null;
let last = 0;
return function(...args) {
let now = +new Date();
if (now - last < interval) {
if (timer) clearTimeout(timer);
timer = setTimeout(() => {
last = now;
fn.apply(this, args);
}, interval);
} else {
last = now;
fn.apply(this, args);
}
};
}
```
使用方式如下:
```javascript
import { debounce, throttle } from '@/utils/debounce-throttle';
// 防抖
const debouncedFn = debounce(() => {
// 函数体
}, 1000);
// 节流
const throttledFn = throttle(() => {
// 函数体
}, 1000);
```
其中,`debounce` 函数用于防抖操作,即在指定时间内,只执行最后一次触发的函数;而 `throttle` 函数用于节流操作,即在指定时间内,每隔一段时间执行一次触发的函数。可以根据实际需求选择不同的函数进行使用。
uniapp 防抖节流
防抖是指在频繁触发某一个事件时,一段时间内或者一定条件下不再触发该事件对应调用的函数。而节流则是在规定时间内仅能触发一次,但到了下一个时间段,也还会继续触发一次事件。
在uniapp中,可以使用以下代码实现防抖和节流的功能:
```javascript
// 防抖
fd() {
let that = this
clearTimeout(this.fdFlag)
this.fdFlag = setTimeout(() => {
that.fdDoing()
that.fdFlag = null
}, 800)
},
fdDoing() {
console.log('防抖事件触发')
},
// 节流
jl() {
let that = this
if (!this.jlFlag) {
return
}
this.jlFlag = false
setTimeout(() => {
that.jlDoing()
that.jlFlag = true
}, 2000)
},
jlDoing() {
console.log('节流事件触发')
}
```
以上代码演示了如何在uniapp中实现防抖和节流功能。在点击按钮时,可以通过调用fd()方法实现防抖功能,只触发最后一次事件。而通过调用jl()方法实现节流功能,每2秒触发一次事件。
阅读全文
相关推荐
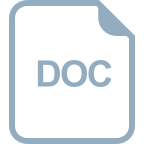
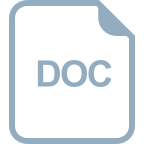
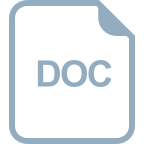



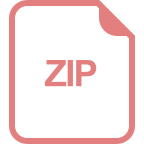
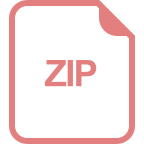
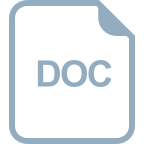







