用pytorch实现在CNN中加入自适应层,然后在这些层加入自适应度量,最后对网络进行 finetune
时间: 2024-03-22 13:37:15 浏览: 21
好的,我来回答你的问题。
首先,自适应层可以通过在PyTorch中定义一个新的层来实现。在这个新的层中,我们需要使用nn.Module类,并实现forward函数。可以参考以下代码:
```
import torch.nn as nn
import torch.nn.functional as F
class AdaptiveLayer(nn.Module):
def __init__(self, in_channels, out_channels):
super(AdaptiveLayer, self).__init__()
self.conv = nn.Conv2d(in_channels, out_channels, kernel_size=3, padding=1)
self.bn = nn.BatchNorm2d(out_channels)
self.relu = nn.ReLU(inplace=True)
self.adaptive_weight = nn.Parameter(torch.ones(out_channels))
def forward(self, x):
x = self.conv(x)
x = self.bn(x)
x = self.relu(x)
x = x * self.adaptive_weight.unsqueeze(-1).unsqueeze(-1)
return x
```
在这个自适应层中,我们定义了一个卷积层、一个批归一化层、一个ReLU激活函数和一个自适应权重。自适应权重是一个可学习的张量,它的形状与输出通道数相同。在前向传递中,我们将自适应权重与输出相乘以对其进行加权。
接下来,我们需要在这些自适应层中加入自适应度量。这可以通过重载backward函数来实现。在这个函数中,我们可以对梯度进行修改以实现自适应度量。具体实现如下:
```
class AdaptiveLayer(nn.Module):
def __init__(self, in_channels, out_channels):
super(AdaptiveLayer, self).__init__()
self.conv = nn.Conv2d(in_channels, out_channels, kernel_size=3, padding=1)
self.bn = nn.BatchNorm2d(out_channels)
self.relu = nn.ReLU(inplace=True)
self.adaptive_weight = nn.Parameter(torch.ones(out_channels))
def forward(self, x):
x = self.conv(x)
x = self.bn(x)
x = self.relu(x)
x = x * self.adaptive_weight.unsqueeze(-1).unsqueeze(-1)
return x
def backward(self, grad_output):
grad_input = grad_output.clone()
adaptive_grad = torch.mean(torch.mean(grad_output, dim=-1), dim=-1).unsqueeze(-1).unsqueeze(-1)
grad_input = grad_input * adaptive_grad * self.adaptive_weight.unsqueeze(-1).unsqueeze(-1)
return grad_input, None, None
```
在这个backward函数中,我们首先使用了clone函数复制了梯度。然后,我们使用自适应权重对梯度进行加权,最后返回修改后的梯度。
最后,我们需要对网络进行finetune。这可以通过定义一个新的模型,它包含我们的自适应层,并使用预训练的模型进行初始化。然后,我们可以使用新模型对数据进行训练。具体实现如下:
```
import torchvision.models as models
pretrained_model = models.resnet18(pretrained=True)
new_model = nn.Sequential(
pretrained_model.conv1,
pretrained_model.bn1,
pretrained_model.relu,
pretrained_model.maxpool,
pretrained_model.layer1,
pretrained_model.layer2,
pretrained_model.layer3,
AdaptiveLayer(256, 512),
AdaptiveLayer(512, 512),
nn.AdaptiveAvgPool2d((1, 1)),
nn.Flatten(),
nn.Linear(512, 10)
)
new_model.train()
# Train the new model
optimizer = torch.optim.SGD(new_model.parameters(), lr=0.001, momentum=0.9)
criterion = nn.CrossEntropyLoss()
for epoch in range(num_epochs):
for i, (images, labels) in enumerate(train_loader):
optimizer.zero_grad()
outputs = new_model(images)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
```
在这个示例中,我们使用了ResNet18作为预训练模型,并定义了一个新模型,它包含了我们的自适应层。然后,我们使用SGD优化器和交叉熵损失对新模型进行训练。
希望这个回答能够帮助到你!
相关推荐
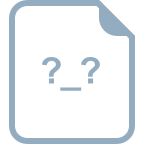
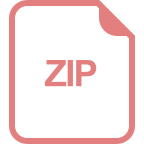














