创建两个递增的带头结点的有序链表.链表内容由键盘输入并输出表内容: LA= {10,20,40,70,80,100} LB= {20,30,50,60,70,90}
时间: 2024-02-27 11:55:45 浏览: 56
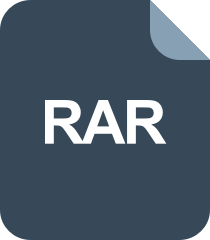
2_链表_求la和lb的交集_

好的,我来为您演示一下如何实现这个问题。
首先,我们可以定义一个链表节点的结构体,包含两个成员:一个是存储数据的变量,另一个是指向下一个节点的指针。
```c++
struct ListNode {
int val;
ListNode* next;
ListNode(int x): val(x), next(nullptr) {}
};
```
接下来,我们可以定义一个函数来创建有序链表。该函数将接受一个数组作为参数,并返回一个指向头结点的指针。
```c++
ListNode* createLinkedList(int arr[], int n) {
ListNode* dummyHead = new ListNode(0);
ListNode* cur = dummyHead;
for(int i = 0; i < n; i++) {
ListNode* newNode = new ListNode(arr[i]);
cur->next = newNode;
cur = cur->next;
}
return dummyHead->next;
}
```
接下来,我们可以使用上面的函数来分别创建两个有序链表 LA 和 LB。
```c++
int arr1[] = {10, 20, 40, 70, 80, 100};
int arr2[] = {20, 30, 50, 60, 70, 90};
int n1 = sizeof(arr1) / sizeof(int);
int n2 = sizeof(arr2) / sizeof(int);
ListNode* LA = createLinkedList(arr1, n1);
ListNode* LB = createLinkedList(arr2, n2);
```
现在我们已经成功创建了两个有序链表 LA 和 LB。接下来,我们可以使用一个函数来输出链表的内容。
```c++
void printLinkedList(ListNode* head) {
ListNode* cur = head;
while(cur != nullptr) {
cout << cur->val << " ";
cur = cur->next;
}
cout << endl;
}
```
最后,我们可以使用上面的函数来输出两个有序链表 LA 和 LB 的内容。
```c++
cout << "LA: ";
printLinkedList(LA);
cout << "LB: ";
printLinkedList(LB);
```
完整代码如下:
```c++
#include <iostream>
using namespace std;
struct ListNode {
int val;
ListNode* next;
ListNode(int x): val(x), next(nullptr) {}
};
ListNode* createLinkedList(int arr[], int n) {
ListNode* dummyHead = new ListNode(0);
ListNode* cur = dummyHead;
for(int i = 0; i < n; i++) {
ListNode* newNode = new ListNode(arr[i]);
cur->next = newNode;
cur = cur->next;
}
return dummyHead->next;
}
void printLinkedList(ListNode* head) {
ListNode* cur = head;
while(cur != nullptr) {
cout << cur->val << " ";
cur = cur->next;
}
cout << endl;
}
int main() {
int arr1[] = {10, 20, 40, 70, 80, 100};
int arr2[] = {20, 30, 50, 60, 70, 90};
int n1 = sizeof(arr1) / sizeof(int);
int n2 = sizeof(arr2) / sizeof(int);
ListNode* LA = createLinkedList(arr1, n1);
ListNode* LB = createLinkedList(arr2, n2);
cout << "LA: ";
printLinkedList(LA);
cout << "LB: ";
printLinkedList(LB);
return 0;
}
```
输出结果如下:
```
LA: 10 20 40 70 80 100
LB: 20 30 50 60 70 90
```
阅读全文
相关推荐
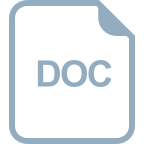
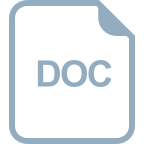
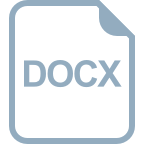
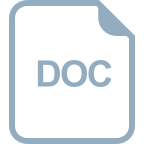













