import requests from bs4 import BeautifulSoup import threading headers = {"User-Agent": 'Mozilla/5.0 (Windows NT 6.1; WOW64) ' 'AppleWebKit/537.36 (KHTML, like Gecko)' 'Chrome/90.0.4430.212 Safari/537.36'} # 定义下载函数 def download(url): response = requests.get(url, headers=headers).text soup = BeautifulSoup(response, features='lxml') src = soup.find_all('img') imagesrc = soup.find_all('img', width="100") for s in imagesrc: with open("{}.jpg".format(s.get('alt')), 'wb') as file: image = requests.get(s.get('src')).content file.write(image) print("正在下载" + s.get('alt') + '.jpg') # 开10个线程下载 threads = [] for x in range(10): url = "https://movie.douban.com/top250?start={}&filter=".format(x * 25) thread = threading.Thread(target=download, args=(url,)) threads.append(thread) thread.start() # 等待所有线程结束 for thread in threads: thread.join()加个显示运行时间的代码
时间: 2023-06-24 08:08:34 浏览: 247
可以在函数开始前加入一个计时器,记录开始时间,函数执行结束后再记录结束时间,并计算时间差,即为函数的运行时间。代码示例如下:
import requests
from bs4 import BeautifulSoup
import threading
import time
headers = {
"User-Agent": 'Mozilla/5.0 (Windows NT 6.1; WOW64) '
'AppleWebKit/537.36 (KHTML, like Gecko)'
'Chrome/90.0.4430.212 Safari/537.36'
}
# 定义下载函数
def download(url):
start_time = time.time() # 记录开始时间
response = requests.get(url, headers=headers).text
soup = BeautifulSoup(response, features='lxml')
src = soup.find_all('img')
imagesrc = soup.find_all('img', width="100")
for s in imagesrc:
with open("{}.jpg".format(s.get('alt')), 'wb') as file:
image = requests.get(s.get('src')).content
file.write(image)
print("正在下载" + s.get('alt') + '.jpg')
end_time = time.time() # 记录结束时间
print("线程 {} 运行时间为:{} 秒".format(threading.current_thread().name, end_time - start_time))
# 开10个线程下载
threads = []
for x in range(10):
url = "https://movie.douban.com/top250?start={}&filter=".format(x * 25)
thread = threading.Thread(target=download, args=(url,), name="Thread-{}".format(x+1))
threads.append(thread)
thread.start()
# 等待所有线程结束
for thread in threads:
thread.join()
阅读全文
相关推荐
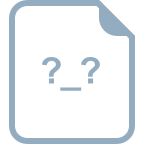
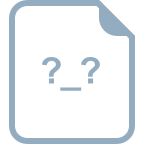
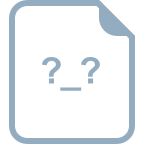


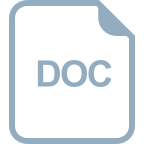
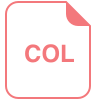
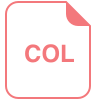
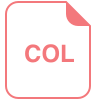
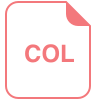
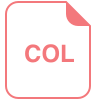
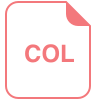
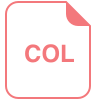
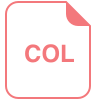
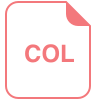
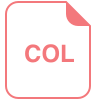
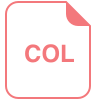
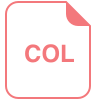
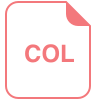
