(InputMismatchException异常)编写一个程序,提示用户读取两个整数,然后显示它们的和。程序应该在输入不正确时提示用户再次读取数值。 输入格式: 输入多组两个数 输出格式: 输出两个数的和 输入样例: 在这里给出一组输入。例如: 1 3 2.0 3 3.0 4 4 5 输出样例: 在这里给出相应的输出。例如: sum = 4 Incorrect input: two integer is required Incorrect input: two integer is required sum = 9 代码长度限制 16 KB 时间限制 400 ms 内存限制 64 MB
时间: 2024-02-16 18:04:23 浏览: 46
下面是代码实现:
```java
import java.util.InputMismatchException;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int num1 = 0, num2 = 0;
while (true) {
try {
num1 = sc.nextInt();
num2 = sc.nextInt();
break;
} catch (InputMismatchException e) {
System.out.println("Incorrect input: two integer is required");
sc.nextLine(); // 清除输入缓冲区中的非整数字符
}
}
System.out.println("sum = " + (num1 + num2));
}
}
```
思路解析:
我们可以通过 `try-catch` 语句块来捕获输入不正确时抛出的 `InputMismatchException` 异常。在 `catch` 语句块中,我们可以输出相应的提示信息,并使用 `Scanner` 类的 `nextLine()` 方法清除输入缓冲区中的非整数字符,以便程序能够读取下一组输入。当读取到两个整数后,程序退出循环,并输出它们的和。
相关推荐
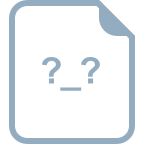
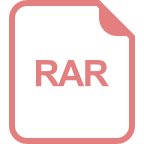
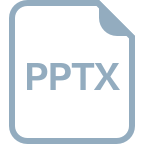













