用c 写一个打开sn_core.elf文件的代码
时间: 2024-03-01 21:49:34 浏览: 19
可以使用C语言标准库中的fopen()函数来打开sn_core.elf文件,示例代码如下:
```c
#include <stdio.h>
int main() {
FILE* fp = fopen("sn_core.elf", "rb"); // 打开文件,以二进制模式读取
if (fp == NULL) {
printf("Failed to open file\n");
return 1;
}
// 文件操作...
fclose(fp); // 关闭文件
return 0;
}
```
在示例代码中,首先使用fopen()函数打开sn_core.elf文件,并以二进制模式读取。如果打开文件失败,则输出错误信息并返回。在文件操作完成后,使用fclose()函数关闭文件。注意,打开文件时需要指定正确的文件路径和文件名,否则会打开失败。
相关问题
/usr/bin/sn_core.elf getstat 2>/dev/null
这是一个 Linux 命令行命令,执行了 /usr/bin/sn_core.elf 可执行文件,并传递了参数 getstat。其中 2>/dev/null 是将 stderr(标准错误输出)重定向到 /dev/null,意味着将错误信息丢弃。该命令可能是用于获取 sn_core.elf 进程的状态信息,并将结果输出到 stdout(标准输出)。
char command[] = "/usr/bin/sn_core.elf getstat 2>/dev/null"; 打印这个数组
好的,您可以使用下面的代码打印这个字符数组:
```c
#include <stdio.h>
int main() {
char command[] = "/usr/bin/sn_core.elf getstat 2>/dev/null";
printf("Command: %s", command);
return 0;
}
```
在上面的代码中,我们定义了一个字符数组 `command`,并初始化为 `"/usr/bin/sn_core.elf getstat 2>/dev/null"`,然后使用 `printf` 函数打印数组的值。输出结果如下:
```
Command: /usr/bin/sn_core.elf getstat 2>/dev/null
```
相关推荐
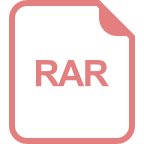

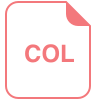

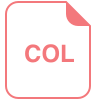









