playfair代码
时间: 2023-07-03 20:21:57 浏览: 94
以下是一个简单的 Playfair 加密算法的 Python 实现:
```python
# Playfair 加密算法 Python 实现
# 构建密钥表格
def build_table(key):
# 去除密钥中的重复字母并转换为大写
key = ''.join(set(key.upper()))
# 去除 J 并将 I 和 J 视为同一个字母
key = key.replace('J', 'I')
# 构建表格
table = []
letters = 'ABCDEFGHIKLMNOPQRSTUVWXYZ'
for letter in key:
if letter not in table:
table.append(letter)
for letter in letters:
if letter not in table:
table.append(letter)
# 将表格转换为 5x5 的矩阵
matrix = [table[i:i+5] for i in range(0, 25, 5)]
return matrix
# 加密函数
def encrypt(plaintext, key):
# 构建密钥表格
table = build_table(key)
# 将明文转换为大写并去除空格
plaintext = plaintext.upper().replace(' ', '')
# 将 I 转换为 J
plaintext = plaintext.replace('I', 'J')
# 如果明文长度为奇数,在末尾添加一个 X
if len(plaintext) % 2 == 1:
plaintext += 'X'
# 逐对加密
ciphertext = ''
for i in range(0, len(plaintext), 2):
# 获取明文中的两个字母
a, b = plaintext[i], plaintext[i+1]
# 获取两个字母在表格中的位置
row1, col1 = divmod(table.index(a), 5)
row2, col2 = divmod(table.index(b), 5)
# 如果两个字母在同一行,将它们分别替换为它们所在行中的下一个字母(循环)
if row1 == row2:
ciphertext += table[row1][(col1+1)%5]
ciphertext += table[row2][(col2+1)%5]
# 如果两个字母在同一列,将它们分别替换为它们所在列中的下一个字母(循环)
elif col1 == col2:
ciphertext += table[(row1+1)%5][col1]
ciphertext += table[(row2+1)%5][col2]
# 否则,用两个字母所在行的另一个字母替换它们
else:
ciphertext += table[row1][col2]
ciphertext += table[row2][col1]
return ciphertext
# 解密函数
def decrypt(ciphertext, key):
# 构建密钥表格
table = build_table(key)
# 将密文转换为大写并去除空格
ciphertext = ciphertext.upper().replace(' ', '')
# 如果密文长度为奇数,在末尾添加一个 X
if len(ciphertext) % 2 == 1:
ciphertext += 'X'
# 逐对解密
plaintext = ''
for i in range(0, len(ciphertext), 2):
# 获取密文中的两个字母
a, b = ciphertext[i], ciphertext[i+1]
# 获取两个字母在表格中的位置
row1, col1 = divmod(table.index(a), 5)
row2, col2 = divmod(table.index(b), 5)
# 如果两个字母在同一行,将它们分别替换为它们所在行中的上一个字母(循环)
if row1 == row2:
plaintext += table[row1][(col1-1)%5]
plaintext += table[row2][(col2-1)%5]
# 如果两个字母在同一列,将它们分别替换为它们所在列中的上一个字母(循环)
elif col1 == col2:
plaintext += table[(row1-1)%5][col1]
plaintext += table[(row2-1)%5][col2]
# 否则,用两个字母所在行的另一个字母替换它们
else:
plaintext += table[row1][col2]
plaintext += table[row2][col1]
return plaintext
```
使用示例:
```python
# 加密
key = 'MONARCHY'
plaintext = 'HELLO WORLD'
ciphertext = encrypt(plaintext, key)
print(ciphertext) # 'DVSOMGZVYGDZ'
# 解密
plaintext = decrypt(ciphertext, key)
print(plaintext) # 'HELXOWORLDX'
```
注意:由于 Playfair 加密算法涉及到矩阵计算,因此代码实现比较复杂。以上代码仅提供一个简单的实现示例,实际使用中可能需要根据具体需求进行修改。
阅读全文
相关推荐






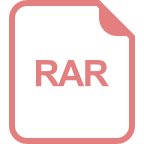


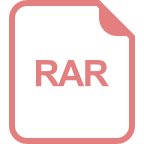





