python Playfair密码代码
时间: 2023-10-13 10:25:44 浏览: 48
下面是一个简单的 Python Playfair 密码实现代码:
```python
from collections import OrderedDict
class PlayfairCipher:
def __init__(self, key):
self.key = key
self.key_matrix = self.generate_matrix(key)
def generate_matrix(self, key):
key = key.upper().replace("J", "I")
key = "".join(OrderedDict.fromkeys(key))
key_matrix = []
for i in range(0, len(key), 5):
key_matrix.append(list(key[i:i+5]))
return key_matrix
def encrypt(self, plaintext):
plaintext = plaintext.upper().replace("J", "I")
plaintext = "".join(OrderedDict.fromkeys(plaintext))
plaintext = plaintext.replace(" ", "")
if len(plaintext) % 2 != 0:
plaintext += "X"
ciphertext = ""
for i in range(0, len(plaintext), 2):
a, b = plaintext[i], plaintext[i+1]
a_pos = self.get_position(a)
b_pos = self.get_position(b)
if a_pos[0] == b_pos[0]:
ciphertext += self.key_matrix[a_pos[0]][(a_pos[1]+1)%5]
ciphertext += self.key_matrix[b_pos[0]][(b_pos[1]+1)%5]
elif a_pos[1] == b_pos[1]:
ciphertext += self.key_matrix[(a_pos[0]+1)%5][a_pos[1]]
ciphertext += self.key_matrix[(b_pos[0]+1)%5][b_pos[1]]
else:
ciphertext += self.key_matrix[a_pos[0]][b_pos[1]]
ciphertext += self.key_matrix[b_pos[0]][a_pos[1]]
return ciphertext
def decrypt(self, ciphertext):
plaintext = ""
for i in range(0, len(ciphertext), 2):
a, b = ciphertext[i], ciphertext[i+1]
a_pos = self.get_position(a)
b_pos = self.get_position(b)
if a_pos[0] == b_pos[0]:
plaintext += self.key_matrix[a_pos[0]][(a_pos[1]-1)%5]
plaintext += self.key_matrix[b_pos[0]][(b_pos[1]-1)%5]
elif a_pos[1] == b_pos[1]:
plaintext += self.key_matrix[(a_pos[0]-1)%5][a_pos[1]]
plaintext += self.key_matrix[(b_pos[0]-1)%5][b_pos[1]]
else:
plaintext += self.key_matrix[a_pos[0]][b_pos[1]]
plaintext += self.key_matrix[b_pos[0]][a_pos[1]]
return plaintext
def get_position(self, letter):
for i, row in enumerate(self.key_matrix):
if letter in row:
return (i, row.index(letter))
raise ValueError(f"{letter} is not in the key matrix")
```
使用示例:
```python
key = "PLAYFAIREXAMPLE"
cipher = PlayfairCipher(key)
plaintext = "Hide the gold in the tree stump"
ciphertext = cipher.encrypt(plaintext)
print(ciphertext) # 输出:BMNDZBXDNABEKUDMUIXMMOUVIF
decrypted_plaintext = cipher.decrypt(ciphertext)
print(decrypted_plaintext) # 输出:HIDETHEGOLDINTHETREXESTUMP
```
相关推荐
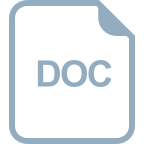













