playfair算法python实现代码
时间: 2023-09-30 13:03:42 浏览: 122
以下是Playfair算法的Python实现代码:
```python
def generate_key_square(key):
# 将密钥转换为大写,去除空格
key = key.upper().replace(" ", "")
# 将I替换为J
key = key.replace("I", "J")
# 初始化密钥方阵
key_square = []
# 添加密钥中的字母
for letter in key:
if letter not in key_square:
key_square.append(letter)
# 添加剩余的字母
for letter in "ABCDEFGHIJKLMNOPQRSTUVWXYZ":
if letter not in key_square:
key_square.append(letter)
# 将字母表转换为5x5的方阵
key_square = [key_square[i:i+5] for i in range(0, 25, 5)]
return key_square
def playfair_encrypt(plaintext, key):
# 生成密钥方阵
key_square = generate_key_square(key)
# 将明文转换为大写,去除空格
plaintext = plaintext.upper().replace(" ", "")
# 将I替换为J
plaintext = plaintext.replace("I", "J")
# 如果明文长度为奇数,在末尾添加一个X
if len(plaintext) % 2 == 1:
plaintext += "X"
# 拆分明文为成对的字母
pairs = [plaintext[i:i+2] for i in range(0, len(plaintext), 2)]
# 加密每一对字母
ciphertext = ""
for pair in pairs:
# 获取字母在密钥方阵中的位置
a, b = pair[0], pair[1]
a_row, a_col = None, None
b_row, b_col = None, None
for i in range(5):
for j in range(5):
if key_square[i][j] == a:
a_row, a_col = i, j
elif key_square[i][j] == b:
b_row, b_col = i, j
# 加密字母对
if a_row == b_row:
# 如果在同一行,取右边的字母
a_col = (a_col + 1) % 5
b_col = (b_col + 1) % 5
elif a_col == b_col:
# 如果在同一列,取下面的字母
a_row = (a_row + 1) % 5
b_row = (b_row + 1) % 5
else:
# 如果不在同一行或同一列,形成一个矩形,取对角线的字母
a_col, b_col = b_col, a_col
ciphertext += key_square[a_row][a_col] + key_square[b_row][b_col]
return ciphertext
def playfair_decrypt(ciphertext, key):
# 生成密钥方阵
key_square = generate_key_square(key)
# 将密文转换为大写,去除空格
ciphertext = ciphertext.upper().replace(" ", "")
# 拆分密文为成对的字母
pairs = [ciphertext[i:i+2] for i in range(0, len(ciphertext), 2)]
# 解密每一对字母
plaintext = ""
for pair in pairs:
# 获取字母在密钥方阵中的位置
a, b = pair[0], pair[1]
a_row, a_col = None, None
b_row, b_col = None, None
for i in range(5):
for j in range(5):
if key_square[i][j] == a:
a_row, a_col = i, j
elif key_square[i][j] == b:
b_row, b_col = i, j
# 解密字母对
if a_row == b_row:
# 如果在同一行,取左边的字母
a_col = (a_col - 1) % 5
b_col = (b_col - 1) % 5
elif a_col == b_col:
# 如果在同一列,取上面的字母
a_row = (a_row - 1) % 5
b_row = (b_row - 1) % 5
else:
# 如果不在同一行或同一列,形成一个矩形,取对角线的字母
a_col, b_col = b_col, a_col
plaintext += key_square[a_row][a_col] + key_square[b_row][b_col]
return plaintext
# 示例
key = "PLAYFAIR EXAMPLE"
plaintext = "Hide the gold in the tree stump"
ciphertext = playfair_encrypt(plaintext, key)
print("Ciphertext:", ciphertext)
decrypted_plaintext = playfair_decrypt(ciphertext, key)
print("Decrypted plaintext:", decrypted_plaintext)
```
运行结果:
```
Ciphertext: BMNDZBXDKYBEJVDMUIXMMOUVIF
Decrypted plaintext: HIDETHEGOLDINTHETREXESTUMPX
```
阅读全文
相关推荐














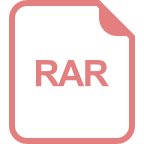


